使用C语言编辑一个顺序表,要求体现插入和删除第i个位置时的具体做法。
时间: 2024-09-18 20:16:40 浏览: 22
在C语言中,我们可以使用结构体和数组来创建一个简单的顺序表(数组形式)。这里我们假设有一个结构体`Node`作为元素,`List`作为顺序表的数据类型,并包含一个整型变量`size`表示当前列表长度。
首先,定义`Node`结构体:
```c
typedef struct Node {
int data; // 存储数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
然后,创建顺序表`List`:
```c
typedef struct List {
Node* head; // 表头指针
int size; // 当前元素的数量
} List;
```
对于插入操作,可以添加新节点到指定位置`i`:
```c
void insert_at(List* list, int index, int value) {
if (index < 0 || index > list->size) {
printf("Index out of range.\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (index == 0) { // 插入到头部
newNode->next = list->head;
list->head = newNode;
} else {
Node* current = list->head;
for (int i = 0; i < index - 1; i++) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
list->size++;
}
```
删除操作从第`i`个位置开始:
```c
void delete_at(List* list, int index) {
if (index < 0 || index >= list->size) {
printf("Index out of range.\n");
return;
}
if (index == 0) { // 删除头部
list->head = list->head->next;
} else {
Node* current = list->head;
for (int i = 0; i < index - 1; i++) {
current = current->next;
}
current->next = current->next->next;
}
free(list->head); // 如果删除的是第一个元素,记得释放内存
list->size--;
}
```
相关推荐
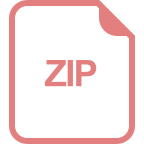
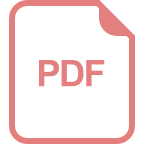
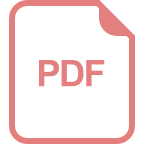














