c#使用opencv实现用鼠标对图片进行选择区域操作代码示例
时间: 2024-09-11 12:17:11 浏览: 52
在C#中使用OpenCV实现用鼠标对图片进行选择区域操作,首先需要确保你已经安装了OpenCV的C#版本,即Emgu CV。然后可以创建一个窗体应用程序,并在其中添加鼠标事件处理函数来实现该功能。以下是一个简单的示例代码,展示了如何使用鼠标来选择图片上的一个区域,并在选择完毕后显示该区域:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using Emgu.CV;
using Emgu.CV.Structure;
using Emgu.CV.CvEnum;
public partial class RegionSelectForm : Form
{
private bool isSelecting = false;
private Rectangle selection = Rectangle.Empty;
private Mat image = new Mat();
private Mat dst = new Mat();
public RegionSelectForm()
{
InitializeComponent();
this.MouseDown += new MouseEventHandler(RegionSelectForm_MouseDown);
this.MouseMove += new MouseEventHandler(RegionSelectForm_MouseMove);
this.MouseUp += new MouseEventHandler(RegionSelectForm_MouseUp);
}
private void RegionSelectForm_MouseDown(object sender, MouseEventArgs e)
{
isSelecting = true;
selection.X = e.X;
selection.Y = e.Y;
selection.Width = 0;
selection.Height = 0;
}
private void RegionSelectForm_MouseMove(object sender, MouseEventArgs e)
{
if (isSelecting)
{
int x = Math.Min(selection.X, e.X);
int y = Math.Min(selection.Y, e.Y);
int width = Math.Abs(selection.X - e.X);
int height = Math.Abs(selection.Y - e.Y);
selection = new Rectangle(x, y, width, height);
this.Invalidate();
}
}
private void RegionSelectForm_MouseUp(object sender, MouseEventArgs e)
{
isSelecting = false;
// 这里可以添加代码来处理选中的区域,例如复制到dst等操作
CvInvoke.cvCopy(image, dst, selection);
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
if (!selection.IsEmpty)
{
// 绘制选中的区域
CvInvoke.cvRectangle(image, selection, new MCvScalar(255, 0, 0), 2);
e.Graphics.DrawImage(image.ToBitmap(), Point.Empty);
}
}
public void LoadImage(string imagePath)
{
image = new Mat(imagePath, ImreadModes.Color);
dst = new Mat(image.Size, DepthType.Cv8U, 3);
this.Size = image.Size;
this.Invalidate();
}
}
// 使用示例
public class Program
{
public static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
RegionSelectForm form = new RegionSelectForm();
form.LoadImage("path_to_your_image.jpg"); // 替换为你的图片路径
Application.Run(form);
}
}
```
以上代码中,`RegionSelectForm` 是一个窗体类,其中包含了鼠标事件处理逻辑以及绘图逻辑。`LoadImage` 方法用于加载图片并初始化窗体的大小。在实际应用中,你可以根据需要来处理选中的区域,例如进行进一步的图像处理或者保存选中区域的图片等。
阅读全文
相关推荐
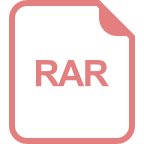
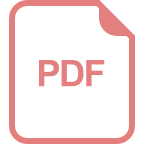
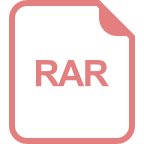

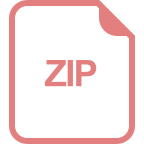
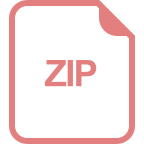
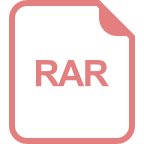
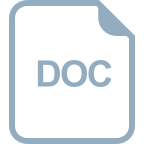
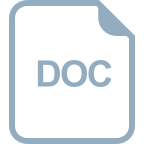
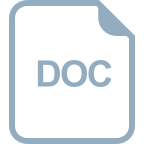
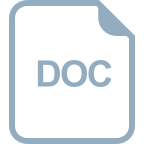
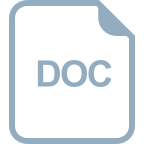
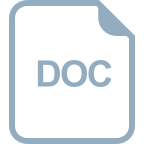
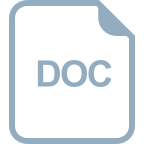
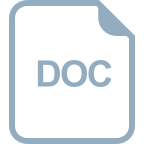
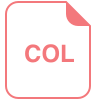



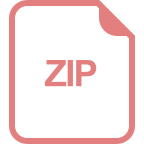