为我写一个用easycaptcha将验证码的图片以base64格式和captchakey发给前端的后端,同时前端做接收
时间: 2024-09-09 14:01:55 浏览: 20
在Java后端使用easycaptcha库生成验证码图片,并将其转换为Base64格式以及生成一个 captchaKey 发送给前端的过程大致如下:
1. 首先,你需要在你的项目中添加easycaptcha依赖。如果你使用Maven,可以在`pom.xml`中添加以下依赖:
```xml
<dependency>
<groupId>com.github.whvcse</groupId>
<artifactId>easycaptcha</artifactId>
<version>版本号</version> <!-- 请替换为最新的版本号 -->
</dependency>
```
2. 在你的后端代码中,你可以创建一个接口来生成验证码并返回给前端。使用`CaptchaUtil`类来生成验证码图片,并使用`Base64`编码来转换图片格式。
```java
import com.github.whvcse.easycaptcha.Captcha;
import com.github.whvcse.easycaptcha.GifCaptcha;
import com.github.whvcse.easycaptcha.CaptchaUtil;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.ByteArrayOutputStream;
@RestController
public class CaptchaController {
@GetMapping("/captcha")
public String getCaptcha(HttpServletRequest request, HttpServletResponse response) throws Exception {
// 创建验证码对象
Captcha captcha = new GifCaptcha(110, 40, 4);
// 生成验证码图片
captcha.createCode();
// 获取验证码文本
String captchaCode = captcha.getCode();
// 将验证码文本存储到session中,用于校验
request.getSession().setAttribute("captchaCode", captchaCode);
// 将验证码图片写到输出流中,这一步是可选的,取决于你是否还需要通过其他方式输出图片
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
captcha.write(response.getOutputStream());
// 将图片字节流转换为Base64编码字符串
String base64Captcha = Base64.getEncoder().encodeToString(outputStream.toByteArray());
// 发送Base64编码的验证码图片和captchaKey到前端
// captchaKey是一个唯一标识,这里使用UUID,实际可以根据需要生成
String captchaKey = java.util.UUID.randomUUID().toString().replaceAll("-", "");
return "{\"captchaKey\":\"" + captchaKey + "\", \"captchaImage\":\"" + base64Captcha + "\"}";
}
}
```
3. 在前端,你可以通过发送AJAX请求到后端的`/captcha`接口来获取Base64编码的验证码图片和captchaKey:
```javascript
fetch('/captcha')
.then(response => response.json())
.then(data => {
document.getElementById('captchaImage').src = 'data:image/png;base64,' + data.captchaImage;
document.getElementById('captchaKey').value = data.captchaKey;
})
.catch(error => {
console.error('Error:', error);
});
```
在这段代码中,`data.captchaImage`是Base64编码的图片字符串,`data.captchaKey`是生成的唯一标识。前端使用`<img>`标签显示验证码图片,并将captchaKey存储在一个输入框中以便之后使用。
相关推荐
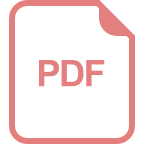
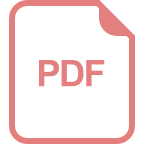















