怎么在python中通过查找字符串来截取字符串部分内容
时间: 2023-07-17 08:07:07 浏览: 48
在Python中,你可以使用字符串的 `find()` 方法或者正则表达式来查找并截取字符串的部分内容。
方法一:使用 `find()` 方法
```python
text = "Hello, world!"
substring = "world"
start_index = text.find(substring)
if start_index != -1:
end_index = start_index + len(substring)
result = text[start_index:end_index]
print(result) # 输出 "world"
else:
print("Substring not found")
```
方法二:使用正则表达式
```python
import re
text = "Hello, world!"
pattern = r"world"
match = re.search(pattern, text)
if match:
result = match.group()
print(result) # 输出 "world"
else:
print("Substring not found")
```
无论是使用 `find()` 方法还是正则表达式,都可以实现在字符串中查找并截取指定内容的功能。你可以根据具体的需求选择合适的方法。
相关问题
python 正则表达 截取字符串中时间
可以使用正则表达式来截取字符串中的时间,下面是一个示例代码:
```python
import re
text = "2021-05-01 12:34:56"
pattern = r"\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}"
match = re.search(pattern, text)
if match:
print("匹配到的时间为:", match.group(0))
else:
print("未找到匹配的时间")
```
这段代码中,我们定义了一个正则表达式 `r"\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}"`,用来匹配类似于 `2021-05-01 12:34:56` 这样的时间格式。然后使用 `re.search()` 函数来在字符串中查找匹配的内容。如果找到了匹配的内容,就使用 `match.group(0)` 来获取匹配到的时间字符串。
python 字符串截取数字部分
你可以使用字符串的切片操作来截取数字部分。假设你的字符串是 `s`,你想截取其中的数字部分,你可以尝试以下代码:
```python
import re
s = "abc123def456"
numbers = re.findall(r'\d+', s)
# 如果你只需要第一个数字,可以使用以下代码
# number = re.search(r'\d+', s).group()
print(numbers) # ['123', '456']
```
在这个例子中,我们使用了正则表达式模块 `re` 的 `findall` 方法来查找字符串中的所有数字部分。如果你只需要第一个数字,你可以使用 `search` 方法。这样,`numbers` 列表中就会包含所有找到的数字部分。
相关推荐
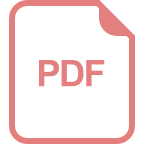
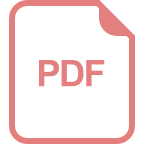
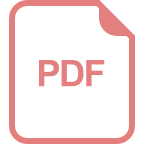












