用python编写一个单顺序表,在命令框中能实现增,删,改,查的功能的代码
时间: 2024-10-28 14:08:02 浏览: 5
在Python中,我们可以使用内置的数据结构列表(List)来模拟一个简单的单顺序表,并通过函数来实现增、删、改、查的操作。这里是一个简单的示例:
```python
class SequentialList:
def __init__(self):
self.data = []
# 插入元素到列表末尾
def insert(self, index, value):
if index < 0 or index > len(self.data):
print("插入位置无效")
else:
self.data.insert(index, value)
# 删除指定索引的元素
def delete(self, index):
if index >= 0 and index < len(self.data):
del self.data[index]
else:
print("删除位置无效")
# 修改指定索引的元素
def update(self, index, new_value):
if index >= 0 and index < len(self.data):
self.data[index] = new_value
else:
print("更新位置无效")
# 查询元素
def search(self, value):
for i, element in enumerate(self.data):
if element == value:
return i
return "未找到该元素"
# 使用示例
list_obj = SequentialList()
list_obj.insert(0, "Apple")
list_obj.insert(1, "Banana")
print(list_obj.search("Apple")) # 输出 0
try:
list_obj.update(2, "Cherry") # 尝试修改不存在的位置
except IndexError:
pass
list_obj.delete(1)
print(list_obj.data) # 输出 ['Apple', 'Cherry']
```
这个例子中,`SequentialList` 类有四个方法分别对应增删改查操作。当你需要对列表进行类似的操作时,可以直接实例化这个类并调用相应方法。
阅读全文
相关推荐
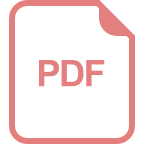
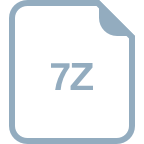
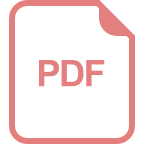
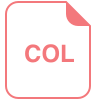
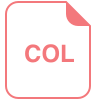
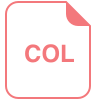
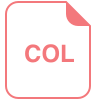
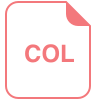
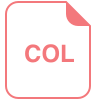
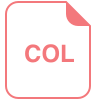
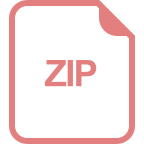
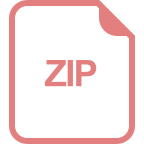