利用MATLAB代码实现图像的分类K-means算法
时间: 2024-09-23 12:15:58 浏览: 57
在MATLAB中实现K-means图像分类算法,通常会分为以下几个步骤:
1. **加载和预处理图像**:
```matlab
% 加载图像数据
img = imread('your_image.jpg');
% 将RGB图像转换为灰度图像,如果需要
gray_img = rgb2gray(img);
% 对图像进行归一化
normalized_img = im2double(gray_img) / 255;
```
2. **将图像转为向量表示**:
```matlab
% 将像素值展平成行向量
pixel_vectors = reshape(normalized_img, [], 1);
```
3. **选择初始质心(中心点)**:
```matlab
% 初始化k个随机质心
k = 5; % 根据类别数设置k值
centroids = rand(k, size(pixel_vectors, 2));
```
4. **迭代K-means过程**:
```matlab
% 设置最大迭代次数和误差阈值
max_iters = 100;
tolerance = 1e-6;
for iter = 1:max_iters
% 分配每个像素到最近的质心
assignments = pdist2(pixel_vectors, centroids, 'euclidean');
clusters = kmeans(pixel_vectors, k, 'InitialCenters', centroids, 'EmptyAction', 'singleton');
% 更新质心
new_centroids = zeros(size(centroids));
for i = 1:k
new_centroids(i,:) = mean(pixel_vectors(clusters == i,:), 1);
end
% 检查停止条件:质心变化小于指定阈值
if norm(new_centroids - centroids, 'fro') < tolerance
break;
end
centroids = new_centroids;
end
```
5. **结果可视化**:
```matlab
% 绘制聚类后的结果
figure;
imagesc(normalized_img);
hold on;
colors = jet(k);
for i = 1:k
cluster_pixels = pixel_vectors(find(clusters == i), :);
plot(cluster_pixels(:,2), cluster_pixels(:,1), '.', 'Color', colors(i,:));
end
hold off;
title(['K-means Clustering Result (k = ' num2str(k) ')']);
```
阅读全文
相关推荐
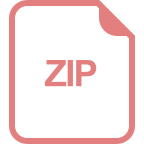
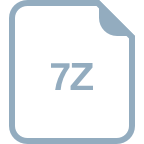
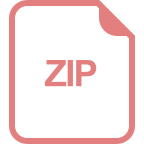

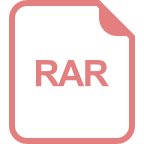
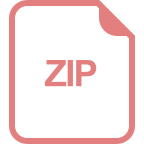
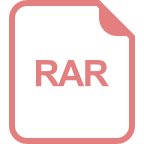
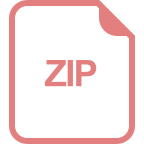
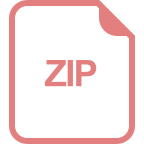
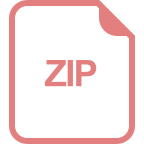
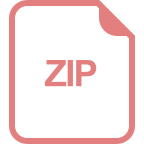
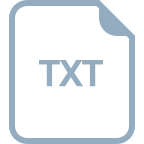
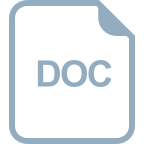
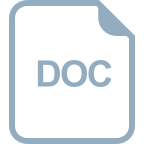
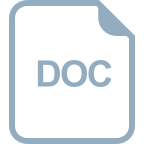
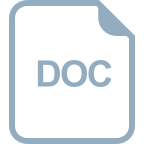
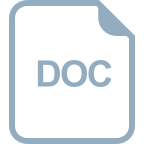