python基于tensorflow欠拟合和过拟合
时间: 2024-01-16 21:16:15 浏览: 89
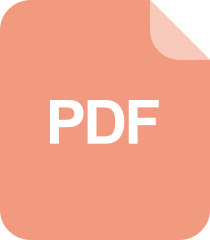
Pytorch之欠拟合和过拟合
以下是基于TensorFlow的欠拟合和过拟合的Python示例:
首先,我们需要导入必要的库和数据集:
```python
import tensorflow as tf
from tensorflow import keras
import numpy as np
# 加载数据集
(x_train, y_train), (x_test, y_test) = keras.datasets.boston_housing.load_data(seed=42)
```
接下来,我们需要对数据进行预处理:
```python
# 标准化数据
mean = x_train.mean(axis=0)
std = x_train.std(axis=0)
x_train = (x_train - mean) / std
x_test = (x_test - mean) / std
```
然后,我们可以定义一个函数来构建模型:
```python
def build_model():
model = keras.Sequential([
keras.layers.Dense(64, activation='relu', input_shape=(x_train.shape[1],)),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(1)
])
optimizer = tf.keras.optimizers.RMSprop(0.001)
model.compile(loss='mse',
optimizer=optimizer,
metrics=['mae'])
return model
```
接下来,我们可以训练模型并绘制训练和验证的损失曲线:
```python
# 构建模型
model = build_model()
# 训练模型
history = model.fit(x_train, y_train, epochs=500, validation_split=0.2, verbose=0)
# 绘制训练和验证的损失曲线
import matplotlib.pyplot as plt
def plot_history(history):
plt.figure()
plt.xlabel('Epoch')
plt.ylabel('Mean Abs Error [$1000]')
plt.plot(history.epoch, np.array(history.history['mae']), label='Train Loss')
plt.plot(history.epoch, np.array(history.history['val_mae']), label = 'Val loss')
plt.legend()
plt.ylim([0,5])
plt.show()
plot_history(history)
```
最后,我们可以使用测试集评估模型的性能:
```python
# 使用测试集评估模型的性能
test_loss, test_mae = model.evaluate(x_test, y_test, verbose=0)
print('Test MAE: ${:,.2f}'.format(test_mae * 1000))
```
阅读全文
相关推荐
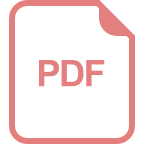
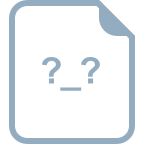
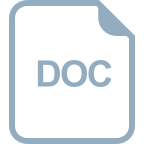
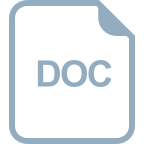
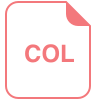
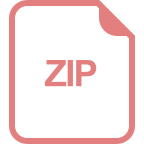
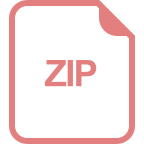
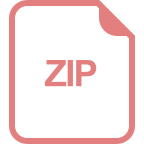
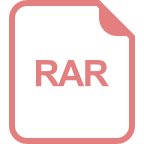
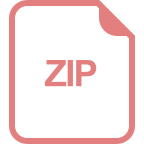
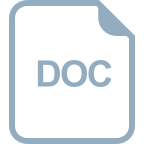
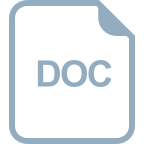
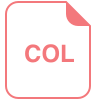
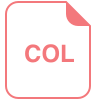
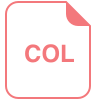
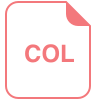