fifo算法代码verilog
时间: 2024-12-26 15:16:06 浏览: 4
### FIFO Algorithm Implementation in Verilog
Below is an example of how a simple First-In-First-Out (FIFO) buffer can be implemented using Verilog:
```verilog
module fifo #(
parameter DEPTH = 8,
parameter WIDTH = 32
)(
input wire clk,
input wire rst_n,
// Write port
input wire wr_en,
input wire [WIDTH-1:0] din,
// Read port
input wire rd_en,
output reg [WIDTH-1:0] dout,
// Status signals
output wire full,
output wire empty
);
reg [DEPTH-1:0][WIDTH-1:0] mem;
reg [$clog2(DEPTH):0] wr_ptr, rd_ptr;
// Full flag generation
wire almost_full = (wr_ptr >= DEPTH - 1);
assign full = almost_full & ~empty;
// Empty flag generation
wire almost_empty = (rd_ptr == wr_ptr);
assign empty = almost_empty;
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
wr_ptr <= 0;
rd_ptr <= 0;
end else begin
if (wr_en && !full)
wr_ptr <= wr_ptr + 1'b1;
if (rd_en && !empty)
rd_ptr <= rd_ptr + 1'b1;
end
end
// Memory write operation
always @(posedge clk) begin
if (wr_en && !full)
mem[wr_ptr] <= din;
end
// Memory read operation with delayed output register
always @(posedge clk) begin
if (rd_en && !empty)
dout <= mem[rd_ptr];
end
endmodule
```
This module defines a generic FIFO structure that supports configurable depth and width parameters. The core functionality includes writing data into memory when `wr_en` is asserted and reading out stored values through `dout`. Control logic ensures proper handling of overflow conditions by monitoring pointer positions relative to the specified buffer size.
For applications requiring stream processing within FPGAs, integrating such FIFO structures alongside HLS streams could enhance overall system throughput while maintaining efficient resource utilization[^2].
阅读全文
相关推荐
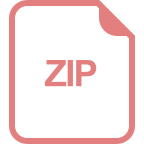
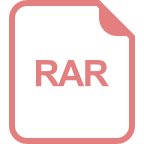
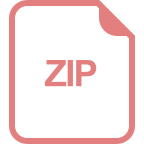
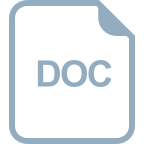
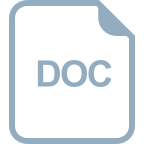
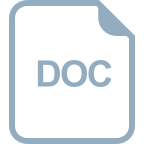

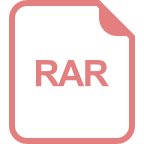
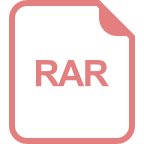
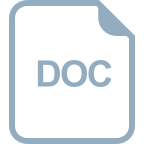
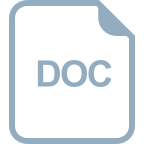
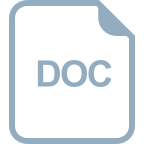
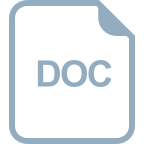
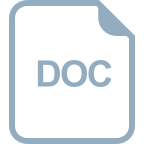
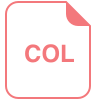
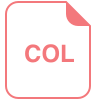
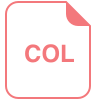
