c++ 数据结构队列
时间: 2024-11-23 08:20:15 浏览: 7
队列(Queue)是一种基本的数据结构,它遵循先进先出(First In First Out, FIFO)的原则。在 C++ 中,标准库提供了一种模板类 `std::queue` 来实现队列。以下是 `std::queue` 的一些关键特性:
1. **元素插入**:`push()` 函数用于在队尾添加元素。
2. **元素删除**:`pop()` 函数用于从队头移除并返回第一个元素,如果队列为空则抛出异常。
3. **查看队头元素**:`front()` 函数返回队头元素,但不会删除它。如果队列为空,此操作也会抛出异常。
4. **查看队尾元素**:由于队列不允许直接访问队尾元素,所以通常使用 `peek()` 或 `rbegin()` 和 `rend()` 进行间接查看,后者是从容器的末尾开始遍历。
5. **迭代器支持**:`std::queue` 提供了迭代器,允许通过 for-each 循环遍历队列元素。
在 C++ 中,你可以这样做来创建和操作队列:
```cpp
#include <queue>
using namespace std;
int main() {
queue<int> q; // 创建一个空的整数队列
// 入队操作
q.push(1);
q.push(2);
q.push(3);
// 出队操作
int frontElement = q.front(); // frontElement 现在等于 1
q.pop();
// 遍历队列
for (auto it = q.begin(); it != q.end(); ++it) {
cout << *it << " ";
}
return 0;
}
```
阅读全文
相关推荐
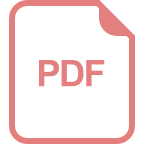
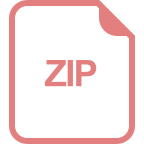
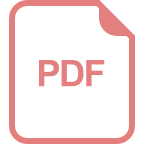
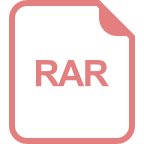
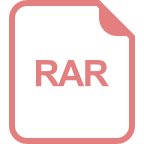
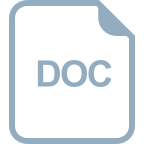
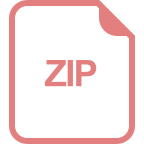
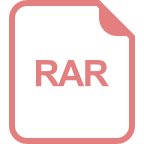
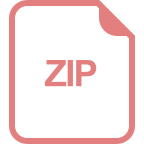
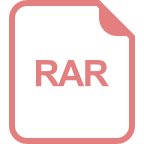
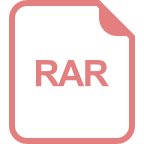
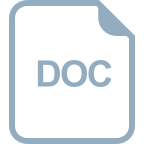
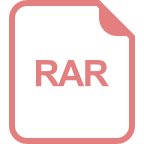
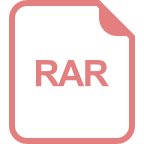
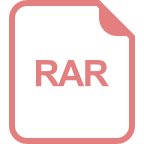
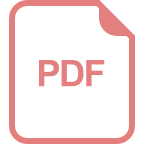
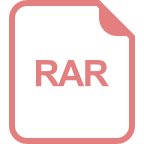