elementui单选框点不动
时间: 2023-09-05 15:14:13 浏览: 157
您好!对于 ElementUI 的单选框无法点击的问题,可能有几个原因导致。以下是一些常见的解决方案:
1. 确保引入了正确的 ElementUI 组件:首先,确保您在代码中正确引入了 ElementUI 的单选框组件。您可以在页面的 `<template>` 标签中使用 `<el-radio>` 标签来创建单选框。
2. 检查组件绑定的值:单选框需要通过 `v-model` 指令与一个变量进行双向绑定。请确保您正确绑定了一个合适的变量,以便正确显示和更新单选框的选中状态。
3. 检查是否禁用了单选框:ElementUI 的单选框组件提供了 `disabled` 属性,用于禁用单选框。如果您设置了该属性为 `true`,则单选框将无法点击。请检查是否意外禁用了单选框。
4. 检查样式和布局问题:有时候,样式和布局问题可能导致单选框无法点击。请确保单选框的父元素没有设置了过大的宽度、高度或溢出隐藏等属性,以免覆盖了单选框导致无法点击。
如果仍然无法解决问题,请提供更多的代码或详细描述,方便我更好地帮助您解决问题。
相关问题
elementUI 单选框如何回显
在 ElementUI 的单选框组件中,我们可以使用 `v-model` 指令来绑定一个数据对象,用于存储当前选中的值。例如:
```html
<template>
<el-radio-group v-model="selected">
<el-radio label="option1">Option 1</el-radio>
<el-radio label="option2">Option 2</el-radio>
<el-radio label="option3">Option 3</el-radio>
</el-radio-group>
</template>
```
在这个例子中,我们将 `selected` 绑定到 `el-radio-group` 的 `v-model` 上,表示当前选中的值。当用户选择其中一个选项时,`selected` 会自动更新为所选选项的 `label` 值。
如果要回显单选框的值,我们只需要将 `selected` 的值设置为我们想要回显的值即可。例如,如果我们想回显 "option2",我们可以在组件的 `data` 中设置 `selected` 的初始值为 "option2":
```js
export default {
data() {
return {
selected: 'option2'
}
}
}
```
这样,当组件初始化时,"Option 2" 的单选框就会被选中,并且 `selected` 的值也会被设置为 "option2"。如果需要动态回显不同的值,只需要在 `selected` 中更新为对应的值即可。
我想用elementUI的多选框组件展示信息,有一个货架信息的下拉菜单,当选中后会吧所有的货架信息以单选框的形式一列一列展示出来,下拉菜单选中的信息单选框上打勾 教我怎么写
好的,你可以按照以下步骤来实现:
1. 在页面中引入 elementUI 的多选框和下拉菜单组件。
2. 定义一个数组 `shelfList` 来存储货架信息的数据,例如:
```javascript
shelfList: [
{ id: 1, name: '货架1' },
{ id: 2, name: '货架2' },
{ id: 3, name: '货架3' },
// ...
]
```
3. 在下拉菜单组件中绑定 `shelfId` 属性来记录当前选中的货架信息的 id 值。
```html
<el-select v-model="shelfId" placeholder="请选择货架信息">
<el-option v-for="item in shelfList" :key="item.id" :value="item.id">{{ item.name }}</el-option>
</el-select>
```
4. 根据选中的货架信息来动态生成单选框组件。
```html
<el-checkbox v-model="checkedShelfIds" :label="item.id" v-for="item in currentShelfList" :key="item.id">{{ item.name }}</el-checkbox>
```
其中,`currentShelfList` 是一个计算属性,用来获取当前选中的货架信息对应的单选框数据,例如:
```javascript
computed: {
currentShelfList() {
const shelf = this.shelfList.find(item => item.id === this.shelfId);
return shelf ? shelf.shelfItems : [];
}
}
```
5. 在 `data` 中定义一个数组 `checkedShelfIds` 来记录当前选中的货架信息的 id 值。
```javascript
data() {
return {
shelfList: [...],
shelfId: null,
checkedShelfIds: []
}
}
```
6. 在单选框组件中绑定 `checkedShelfIds` 数组,用来记录选中的货架信息。
```html
<el-checkbox v-model="checkedShelfIds" :label="item.id" v-for="item in currentShelfList" :key="item.id">{{ item.name }}</el-checkbox>
```
7. 在下拉菜单组件的 `change` 事件中重新计算当前选中的货架信息,并清空选中的单选框信息。
```javascript
methods: {
handleShelfChange() {
this.checkedShelfIds = [];
}
}
```
最终的代码实现大致如下:
```html
<template>
<div>
<el-select v-model="shelfId" placeholder="请选择货架信息" @change="handleShelfChange">
<el-option v-for="item in shelfList" :key="item.id" :value="item.id">{{ item.name }}</el-option>
</el-select>
<div v-if="currentShelfList.length">
<el-checkbox v-model="checkedShelfIds" :label="item.id" v-for="item in currentShelfList" :key="item.id">{{ item.name }}</el-checkbox>
</div>
</div>
</template>
<script>
export default {
data() {
return {
shelfList: [
{ id: 1, name: '货架1', shelfItems: [{ id: 1, name: '货架1-1' }, { id: 2, name: '货架1-2' }, { id: 3, name: '货架1-3' }] },
{ id: 2, name: '货架2', shelfItems: [{ id: 4, name: '货架2-1' }, { id: 5, name: '货架2-2' }, { id: 6, name: '货架2-3' }] },
{ id: 3, name: '货架3', shelfItems: [{ id: 7, name: '货架3-1' }, { id: 8, name: '货架3-2' }, { id: 9, name: '货架3-3' }] },
],
shelfId: null,
checkedShelfIds: []
}
},
computed: {
currentShelfList() {
const shelf = this.shelfList.find(item => item.id === this.shelfId);
return shelf ? shelf.shelfItems : [];
}
},
methods: {
handleShelfChange() {
this.checkedShelfIds = [];
}
}
}
</script>
```
阅读全文
相关推荐
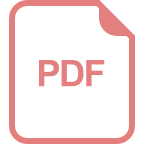
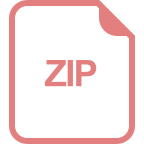
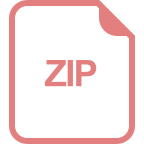
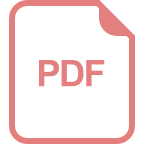
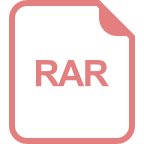
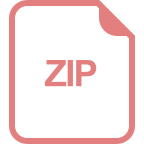
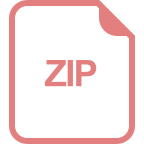
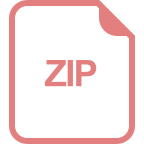
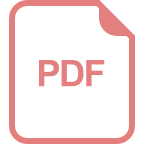
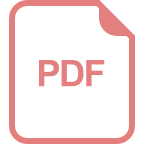
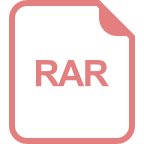
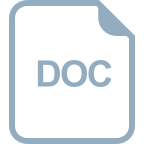
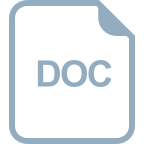
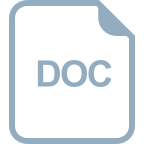
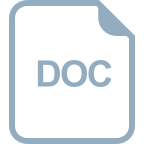
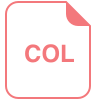