c语言中 删除顺序表中重复元素的代码完整版本
时间: 2024-09-17 07:09:29 浏览: 92
在C语言中,删除顺序表(通常指单链表)中的重复元素,你可以创建一个辅助函数来检查新节点是否已经存在于列表中,如果存在则跳过,否则添加到列表中。以下是一个简单的示例,假设我们有一个`struct Node`表示链表的节点,其中包含`data`成员存储数据,`next`成员指向下一个节点:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 辅助函数,用于查找是否存在重复
int isDuplicate(Node* list, int value) {
while (list != NULL) {
if (list->data == value)
return 1; // 找到了重复值,返回1
list = list->next;
}
return 0; // 没有找到重复值,返回0
}
// 创建新节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 删除重复元素并更新链表
void deleteDuplicates(Node** head) {
if (*head == NULL)
return;
Node* current = *head;
Node* temp = *head;
while (current != NULL) {
if (isDuplicate(temp, current->data)) { // 如果当前节点有重复
Node* nextTemp = temp->next; // 保存下一个节点
free(current); // 释放当前节点内存
temp->next = current->next; // 跳过重复节点
current = nextTemp; // 更新当前节点指针
} else {
temp = temp->next; // 没有重复,继续遍历
current = current->next;
}
}
}
// 测试函数
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
Node* list = createNode(1);
list = createNode(2);
list = createNode(3);
list = createNode(4);
list = createNode(5); // 添加重复的5
deleteDuplicates(&list);
printf("List with duplicates removed: ");
printList(list);
return 0;
}
```
阅读全文
相关推荐











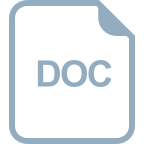
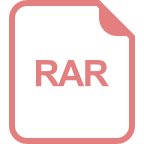





