如何在Python中处理C库函数发送的unsigned char指针sbuffer作为动态参数?
时间: 2024-11-22 18:44:06 浏览: 0
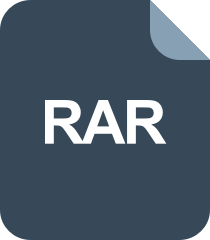
C语言库函数速查手册 C语言库函数速查手册

在Python中处理C库函数并传递unsigned char类型的指针作为动态参数通常涉及两个步骤:C接口的编写和Python与C之间的数据交换。
1. **C接口**:
- 首先,在C语言层面,你需要将接收到的`unsigned char* sbuffer`转换成C能够接受的数据结构。这可能是一个`void*`、`char*`数组或者其他形式的内存缓冲区。如果函数需要处理特定长度的字符串,记得保存那个长度信息。
```c
#include <string.h>
typedef struct {
size_t length; // 字符串长度
unsigned char *data; // 字符数组指针
} BufferStruct;
extern int c_function(BufferStruct *buf);
```
2. **Python绑定**:
- 使用Python的C API(ctypes模块)可以方便地创建Python类型和C类型的桥梁。首先,定义一个Python类表示`BufferStruct`:
```python
from ctypes import Structure, c_char_p, c_size_t
class BufferStruct(Structure):
_fields_ = [('length', c_size_t), ('data', c_char_p)]
_lib = cdll.LoadLibrary('your_c_library.so') # 替换为实际的C库路径
_lib.c_function.argtypes = [BufferStruct]
_lib.c_function.restype = int
```
3. **Python调用**:
- 在Python脚本中,你可以创建一个`BufferStruct`实例,并设置`data`成员为你的`unsigned char`指针和相应的长度:
```python
sbuffer = b"Hello, Python!"
buffer_length = len(sbuffer)
buf = BufferStruct(length=buffer_length, data=sbuffer)
result = _lib.c_function(buf) # 调用C函数,注意处理返回值
```
4. **结果处理**:
- C函数处理完`sbuffer`后,你可以在Python中根据需要处理`result`。
阅读全文
相关推荐
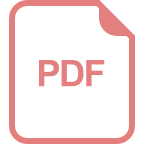
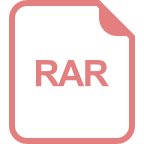
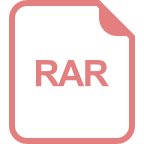
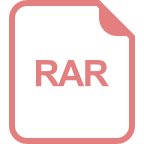
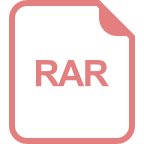
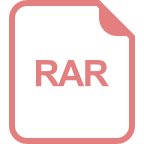
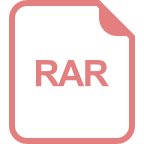
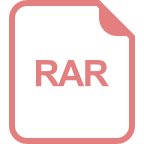
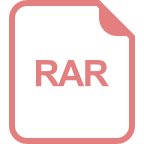

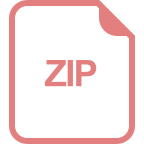
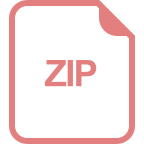