定义一个yml配置文件,描述员工的基本信息,包括姓名(字符串)、出生年月(日期)、兴趣爱好(列表)、性别(字符串)等信息。 请用上述yml配置文件中的的员工属性值定义员工类,选择合适的方法实现配置文件属性值的注入.使用springboot知识完成
时间: 2024-09-30 09:11:20 浏览: 31
在YAML (YAML Ain't Markup Language) 配置文件中,我们可以这样描述一个员工的信息:
```yaml
employee:
name: 张三
birth_date: !date 1990-01-01
interests:
- 篮球
- 游泳
gender: 男
```
在这个例子中,`name`是一个字符串,`birth_date`是一个日期格式的字符串,`interests`是一个包含字符串元素的列表,`gender`也是一个字符串。
为了将这些属性值注入到Spring Boot应用中的Employee类,我们首先需要创建一个Employee实体类:
```java
import org.springframework.core.convert.converter.Converter;
import org.springframework.format.annotation.DateTimeFormat;
import java.util.Date;
import java.util.List;
public class Employee {
private String name;
@DateTimeFormat(pattern = "yyyy-MM-dd")
private Date birthDate;
private List<String> interests;
private String gender;
// getters and setters
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public Date getBirthDate() { return birthDate; }
public void setBirthDate(Date birthDate) { this.birthDate = birthDate; }
public List<String> getInterests() { return interests; }
public void setInterests(List<String> interests) { this.interests = interests; }
public String getGender() { return gender; }
public void setGender(String gender) { this.gender = gender; }
// 如果需要自定义日期转换器,可以提供一个Converter实现
public static class BirthDateConverter implements Converter<String, Date> {
@Override
public Date convert(String value) {
return DateTimeFormatter.ofPattern("yyyy-MM-dd").parse(value);
}
}
}
```
然后,在Spring Boot的配置中,你可以使用`@ConfigurationProperties`注解来自动绑定YAML配置到这个类:
```java
import org.springframework.boot.context.properties.ConfigurationProperties;
import java.util.List;
@ConfigurationProperties(prefix = "employee")
public class EmployeeProperties {
private String name;
private Date birthDate;
private List<String> interests;
private String gender;
// ... setter methods and getters
public static class BirthDateConverter implements Converter<String, Date> {
// same as above
}
}
```
最后,在启动类中启用这个配置:
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public Employee employeeFromConfig(EmployeeProperties properties) {
Employee employee = new Employee();
employee.setName(properties.getName());
employee.setBirthDate(BirthDateConverter.convert(properties.getBirthDate()));
employee.setInterests(properties.getInterests());
employee.setGender(properties.getGender());
return employee;
}
}
```
这样,当应用程序运行时,Spring Boot会读取YML配置并将其注入到Employee实例中。
阅读全文
相关推荐
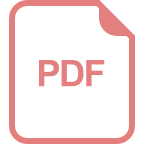
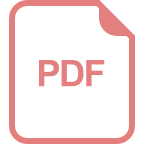
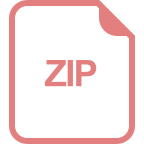








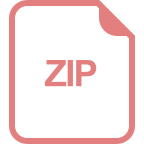
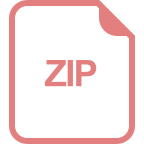
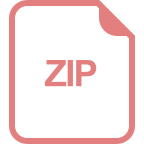
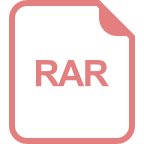
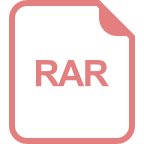
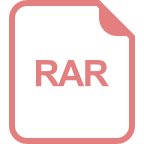
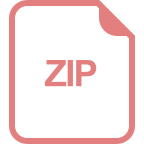