如何修正将'int*'转换为'const char*'时出现的编译错误?
时间: 2024-11-03 15:16:09 浏览: 11
当你尝试将 `int*` 类型转换为 `const char*` 类型时,如果原始指针指向的是整数变量而非字符数组或者字符串,这就会导致编译错误,因为它们的内存结构并不兼容。
要修正这种错误,你需要确保:
1. **检查指针内容**:`int*` 指向的是可以解读为字节的内存地址,如果它实际上是有效的 `char` 或 `wchar_t` 字符数据,你可以直接将其视为 `const char*`。
2. **转换前确保是字符串**:如果你确信 `int*` 指向的是一个字符串常量(如ASCII或Unicode编码的字符数组),你可以先将其复制到一个新的 `char` 数组中,然后使用这个新的 `char*` 引用。
3. **显式转换**:在C++中,你可以通过类型转换运算符 `static_cast` 来强行转换,但需要清楚这样做可能会丢失原有类型的某些特性,除非你知道原始值代表的是字符串。
例如:
```cpp
// 假设int指针p实际上是一个字符串常量
const int* pInt = ...;
size_t len = sizeof(int) * CHAR_BIT; // 通常为4或8位宽的长度
char* str = new char[len];
memcpy(str, pInt, len); // 将整数值复制成字符数组
const char* strConst = str; // 现在strConst指向了一个const char*
delete[] str; // 别忘了释放内存
```
相关问题
const char* GetDeviceMAC(unsigned int i) unsigned int GetNumberOfAttribute(const char* pDeviceMac) const char* GetAttributeName(const char* pDeviceMac, unsigned int i) 的python ctypes调用
你可以使用Python的ctypes库来调用C++代码中的这些函数。首先,你需要将C++代码编译成共享库(.so文件),然后在Python中使用ctypes库加载该共享库并调用其中的函数。下面是一个简单的示例:
1. 创建一个C++源文件,比如"device.cpp",其中包含你提供的这些函数的实现。
```cpp
// device.cpp
const char* GetDeviceMAC(unsigned int i) {
// 实现函数逻辑
// ...
}
unsigned int GetNumberOfAttribute(const char* pDeviceMac) {
// 实现函数逻辑
// ...
}
const char* GetAttributeName(const char* pDeviceMac, unsigned int i) {
// 实现函数逻辑
// ...
}
```
2. 将C++代码编译成共享库。在终端中运行以下命令:
```bash
g++ -shared -o device.so -fPIC device.cpp
```
这将生成一个名为"device.so"的共享库文件。
3. 在Python中使用ctypes库加载共享库并调用函数。创建一个Python脚本,比如"main.py",并添加以下内容:
```python
# main.py
import ctypes
# 加载共享库
device = ctypes.CDLL('./device.so')
# 获取函数签名
device.GetDeviceMAC.restype = ctypes.c_char_p
device.GetDeviceMAC.argtypes = [ctypes.c_uint]
device.GetNumberOfAttribute.restype = ctypes.c_uint
device.GetNumberOfAttribute.argtypes = [ctypes.c_char_p]
device.GetAttributeName.restype = ctypes.c_char_p
device.GetAttributeName.argtypes = [ctypes.c_char_p, ctypes.c_uint]
# 调用函数
mac = device.GetDeviceMAC(0)
num_attributes = device.GetNumberOfAttribute(mac)
attribute_name = device.GetAttributeName(mac, 0)
# 打印结果
print("MAC:", mac)
print("Number of attributes:", num_attributes)
print("Attribute name:", attribute_name)
```
确保将共享库文件"device.so"与Python脚本"main.py"放在同一目录下。然后在终端中运行以下命令:
```bash
python main.py
```
这将调用C++函数并打印结果。
请注意,你需要根据实际情况修改函数的参数类型和返回类型。此示例假设函数返回的是C风格的字符串(char*),并接受无符号整数作为参数。你可能还需要进行其他的类型转换和错误处理,这取决于你的具体需求。
int strcmp(const char*,const char*)无法从char转换为const
这个错误可能是因为您在函数中传递了一个 `char` 类型的参数,但是函数的参数类型是 `const char*`。这种错误通常是由于函数参数类型与传递的参数类型不匹配导致的。
例如,如果您有以下函数声明:
```
int strcmp(const char* str1, const char* str2);
```
那么您调用该函数时必须传递两个指向 `const char` 类型的指针作为参数。如果您传递一个 `char` 类型的参数,编译器将会报错。
为了解决这个问题,您可以将 `char` 类型的参数转换为 `const char*` 类型,例如:
```
char c = 'a';
int result = strcmp(&c, "hello"); // 将字符 'a' 转换为指向字符数组的指针
```
或者,您也可以修改函数声明,将参数类型更改为 `char` 类型,例如:
```
int my_strcmp(char str1, char str2);
```
这样,您就可以传递 `char` 类型的参数,而不会出现编译错误了。
阅读全文
相关推荐
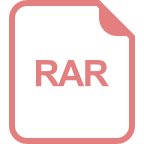
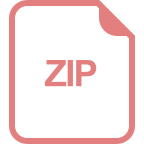
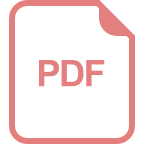














