C++ 构建一个结构体,把数据传入到结构体。然后构建一个类,把结构体里面的数据传入类,最后通过类把文本文件里的东西输出出来。 相关文本文件已经上传到数据结构的群里
时间: 2024-09-10 20:30:45 浏览: 34
在C++中,你可以首先创建一个结构体来存储你需要的数据,然后定义一个类来封装这个结构体,以便于操作。假设我们有如下的结构体`Student`用于存储学生的姓名、年龄和分数:
```cpp
#include <fstream>
#include <string>
// 结构体 Student
struct Student {
std::string name;
int age;
double score;
};
```
接着我们可以创建一个`FileProcessor`类,它接受`Student`作为内部数据,并提供一个方法来读取文件内容并将学生信息插入到结构体内:
```cpp
class FileProcessor {
private:
Student studentData;
public:
void processFile(const std::string& fileName) {
std::ifstream inputFile(fileName);
if (inputFile.is_open()) {
inputFile >> studentData.name >> studentData.age >> studentData.score;
inputFile.close();
outputStudentData(studentData); // 输出方法
} else {
std::cerr << "Failed to open the file." << std::endl;
}
}
// 输出结构体的内容
void outputStudentData(const Student& data) {
std::cout << "Name: " << data.name << ", Age: " << data.age << ", Score: " << data.score << std::endl;
}
};
```
要使用这个类,你可以这样做:
```cpp
int main() {
FileProcessor processor;
processor.processFile("students.txt"); // 假设students.txt中有数据
return 0;
}
```
在这个例子中,`processFile`方法会读取指定的文本文件中的数据,然后通过`outputStudentData`方法将其打印出来。
相关推荐
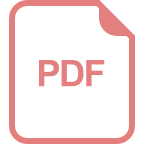
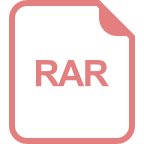
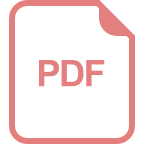














