C语言编程实现:编程实现:某航空公司规定在旅游旺季(7~9 月),票价九折优惠;在旅游淡季(3~5 月、11 月),票价六折优惠;其他月份,票价八折优惠。根据输入的月份及票价,计算并输出应付金额。
时间: 2024-10-20 17:14:25 浏览: 87
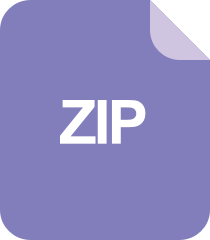
C语言编程实战:成绩管理系统.zip
在C语言中,我们可以创建一个函数来处理这种条件折扣计算。这里是一个简单的实现:
```c
#include <stdio.h>
#include <stdbool.h> // 引入bool类型用于判断季节
// 定义票价函数
double calculate_ticket_price(int month) {
double base_price = 100.0; // 假设基础票价为100元
bool is_peak_season;
// 根据月份判断季节和打折情况
if (month >= 7 && month <= 9) { // 旺季
is_peak_season = true;
} else if (month >= 3 && month <= 5 || month == 11) { // 淡季
is_peak_season = false;
} else { // 其他月份
is_peak_season = false;
}
// 根据季节给予相应折扣
if (is_peak_season) {
return base_price * 0.9; // 九折
} else if (!is_peak_season) {
return base_price * 0.6; // 六折
} else {
return base_price * 0.8; // 八折
}
}
int main() {
int input_month;
printf("请输入月份(1-12): ");
scanf("%d", &input_month);
double ticket_cost = calculate_ticket_price(input_month);
printf("应付金额为 %.2f 元。\n", ticket_cost); // 输出到两位小数
return 0;
}
```
在这个程序中,我们首先获取用户的月份输入,然后通过`calculate_ticket_price`函数根据月份判断是哪一类型的季节,并给出相应的折扣。注意这只是一个基本示例,实际应用中可能需要更复杂的错误检查。
阅读全文
相关推荐
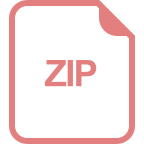
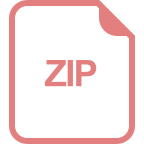
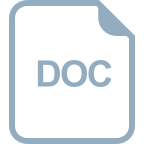
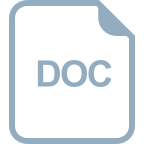
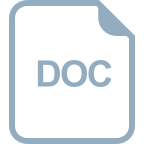
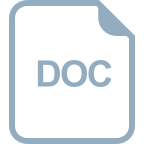
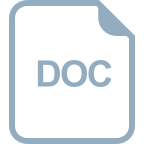
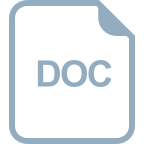
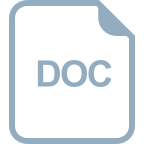
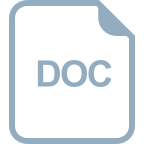
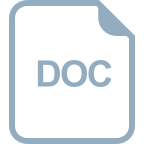
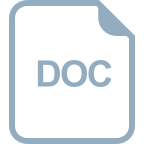
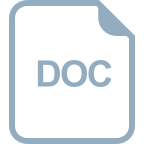
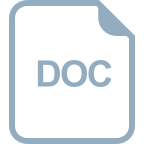
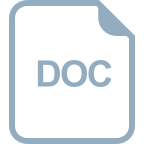
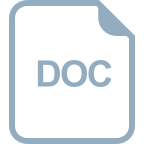
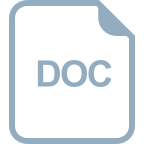
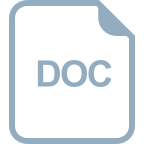