编写Java程序,声明三个不同类型的变量,输出它们进行加减乘除之后的结果值。
时间: 2024-09-21 14:08:58 浏览: 10
在Java中,你可以使用`Object`类型作为父类,并通过继承创建不同的子类来演示多态。这里是一个简单的例子:
```java
// 定义一个Number接口
interface Number {
double add(double other);
double subtract(double other);
double multiply(double other);
double divide(double other);
}
// 实现数字类型接口的抽象类,比如整数、浮点数等
class IntegerImpl implements Number {
private int value;
// 实现接口方法
@Override
public double add(double other) {
return (double) this.value + other;
}
@Override
public double subtract(double other) {
return (double) this.value - other;
}
@Override
public double multiply(double other) {
return this.value * other;
}
@Override
public double divide(double other) {
if (other != 0) {
return this.value / other;
} else {
throw new ArithmeticException("Cannot divide by zero");
}
}
}
class FloatImpl implements Number {
private float value;
// 类似于IntegerImpl,实现相同的方法
//...
}
public class Main {
public static void main(String[] args) {
// 创建IntegerImpl对象
Number integer = new IntegerImpl();
integer.value = 5; // 假设是整数5
// 创建FloatImpl对象
Number floatNum = new FloatImpl();
floatNum.value = 5.5f; // 假设是浮点数5.5
// 输出操作结果
System.out.println("Integer addition: " + integer.add(2));
System.out.println("Integer subtraction: " + integer.subtract(1));
System.out.println("Integer multiplication: " + integer.multiply(2));
// 类型转换并输出
System.out.println("Multiplication with type cast: " + ((IntegerImpl)floatNum).multiply(2));
// 尝试除法
try {
System.out.println("Division: " + integer.divide(floatNum.value));
} catch (ArithmeticException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
```
在这个示例中,虽然`integer`和`floatNum`都是`Number`类型的引用,但由于它们指向的是不同的子类实例,所以在运行时会根据实际类型调用对应的操作。
相关推荐
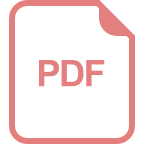
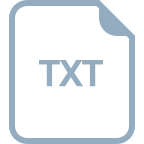
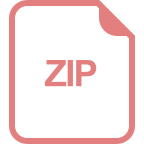














