设计一个特殊的数字列表,能够直接对其中的元素进行批量的四则运算
时间: 2024-10-17 19:10:40 浏览: 24
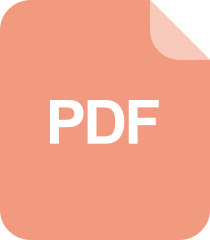
003对单一数据集处理.pdf
设计这样一个特殊数字列表,可以考虑使用Python的内置数据结构`List`或者自定义一个类,结合一些计算函数来实现。我们可以创建一个名为`CalculatorList`的类,它包含以下几个关键属性和方法:
1. **元素存储**:每个元素应该是支持基本算术操作的对象,比如整数、浮点数,或者是实现了加减乘除等运算的自定义类实例。
```python
class CalculatorElement:
def __init__(self, value):
self.value = value
class CalculatorList(list):
```
2. **初始化**:在初始化`CalculatorList`时,可以直接传入一系列`CalculatorElement`对象。
```python
def __init__(self, elements=None):
if elements is not None:
for element in elements:
if not isinstance(element, CalculatorElement):
raise ValueError("Elements must be CalculatorElement instances")
super().__init__(elements)
```
3. **四则运算方法**:添加对所有元素进行批量四则运算的方法,如`add`, `subtract`, `multiply`, 和 `divide`。
```python
class CalculatorList:
# ...
def add(self, other):
return [a + b.value for a, b in zip(self, other)]
def subtract(self, other):
return [a - b.value for a, b in zip(self, other)]
def multiply(self, other):
return [a * b.value for a, b in zip(self, other)]
def divide(self, other):
result = [a / b.value for a, b in zip(self, other) if b.value != 0]
return result
```
4. **验证运算对象**:为了保证运算的合法性,可以在执行运算前检查另一个列表是否也是`CalculatorList`或具有正确的元素类型。
现在,你可以创建这样的列表,并对其进行批量四则运算:
```python
# 创建一个CalculatorList实例
calc_list = CalculatorList([10, 20, 30])
other_list = [5, 15]
# 执行批量运算
sum_result = calc_list.add(other_list)
difference = calc_list.subtract(other_list)
# 类似地,还可以使用multiply()和divide()
```
阅读全文
相关推荐
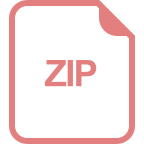
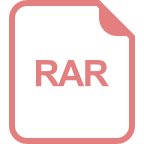


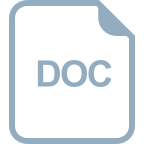
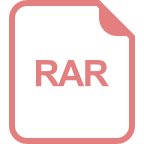
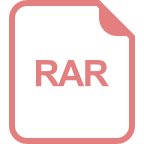
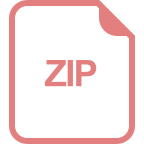
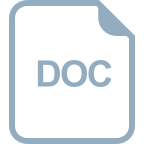
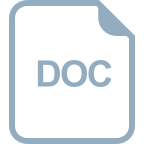
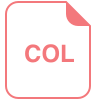
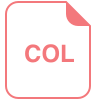
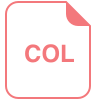
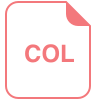
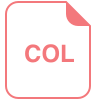
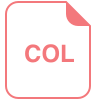
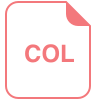
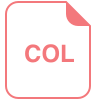