springboot整合grpc
时间: 2023-09-23 08:07:30 浏览: 116
要在Spring Boot中整合gRPC,可以按照以下步骤进行操作:
1. 首先,在pom.xml文件中添加gRPC和protobuf的依赖。例如:
```xml
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty-shaded</artifactId>
<version>1.40.1</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-protobuf</artifactId>
<version>1.40.1</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-stub</artifactId>
<version>1.40.1</version>
</dependency>
```
2. 定义一个gRPC服务,使用Protocol Buffers(protobuf)编写服务定义。例如,创建一个名为`HelloWorld.proto`的文件,定义一个简单的HelloWorld服务:
```protobuf
syntax = "proto3";
package com.example.grpc;
service HelloWorldService {
rpc sayHello (HelloRequest) returns (HelloResponse);
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
```
3. 使用protobuf插件生成Java类文件。可以通过在pom.xml中配置protobuf插件来实现。例如:
```xml
<build>
<plugins>
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.6.1</version>
<configuration>
<protocArtifact>com.google.protobuf:protoc:3.17.3:exe:${os.detected.classifier}</protocArtifact>
<pluginId>grpc-java</pluginId>
<pluginArtifact>io.grpc:protoc-gen-grpc-java:1.40.1:exe:${os.detected.classifier}</pluginArtifact>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
```
运行`mvn clean install`命令,将会生成Java类文件。
4. 创建实现gRPC服务的类。例如,创建一个名为`HelloWorldServiceImpl`的类,实现HelloWorldService:
```java
import io.grpc.stub.StreamObserver;
import com.example.grpc.HelloRequest;
import com.example.grpc.HelloResponse;
import com.example.grpc.HelloWorldServiceGrpc;
public class HelloWorldServiceImpl extends HelloWorldServiceGrpc.HelloWorldServiceImplBase {
@Override
public void sayHello(HelloRequest request, StreamObserver<HelloResponse> responseObserver) {
String message = "Hello, " + request.getName() + "!";
HelloResponse response = HelloResponse.newBuilder().setMessage(message).build();
responseObserver.onNext(response);
responseObserver.onCompleted();
}
}
```
5. 在Spring Boot应用程序中配置gRPC服务。例如,创建一个名为`GrpcServerConfiguration`的类,配置gRPC服务器:
```java
import org.lognet.springboot.grpc.GRpcService;
import com.example.grpc.HelloWorldServiceGrpc;
@Configuration
public class GrpcServerConfiguration {
@Bean
public Server grpcServer() {
return ServerBuilder.forPort(9090).addService(new HelloWorldServiceImpl()).build();
}
@GRpcService
public HelloWorldServiceGrpc.HelloWorldServiceImplBase helloWorldService() {
return new HelloWorldServiceImpl();
}
}
```
6. 启动Spring Boot应用程序,并在9090端口上监听gRPC请求。你可以使用gRPC客户端与服务进行通信了。
阅读全文
相关推荐

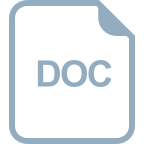
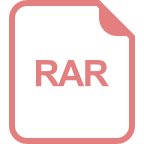
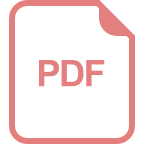
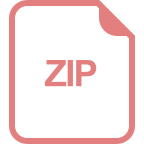
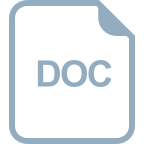
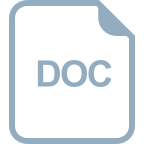

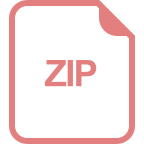
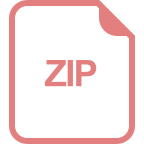
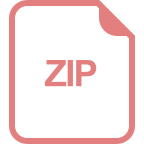
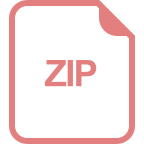
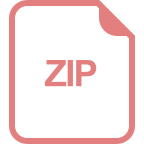
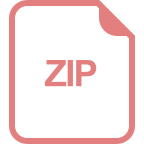
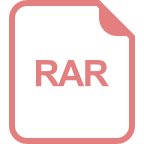
