uniapp开发app时,使用input原生组件如何对键盘上面的按钮文字进行修改,解决方法以及详细代码
时间: 2024-12-08 12:16:33 浏览: 78
在UniApp开发中,如果你想更改`<input>`原生组件键盘上按钮的文字,比如搜索、取消等,这通常涉及到定制输入框的行为,而非直接修改原生键盘。UniApp提供了一些自定义组件属性,可以间接影响输入框的行为,但是无法直接控制键盘的文本。
不过,你可以通过监听`focus`和`confirm`事件,在事件处理函数中动态设置输入框的placeholder属性来模拟这个效果。当用户点击搜索按钮时,你可以在`confirm`事件中改变输入框的提示信息。这里是一个简单的示例:
```html
<template>
<view>
<input placeholder="默认搜索" @focus="onFocus" @confirm="onConfirm" />
</view>
</template>
<script>
export default {
methods: {
onFocus() {
this.placeholder = '正在搜索...'; // 当焦点进入时显示搜索状态
},
onConfirm() {
this.placeholder = '默认搜索'; // 用户确认后恢复到默认状态
},
},
};
</script>
```
注意:这种方法并不是修改原生键盘上的文字,而是改变了输入框本身的提示。如果你需要更复杂的交互,可能需要借助第三方插件或者调用原生API,但这超出了uni-app的基础功能范围。
相关问题
在使用UniApp开发app应用时,如何针对原生input组件修改键盘上按钮的文字(如保存、取消等),能否提供详细的实现步骤及相应的代码示例?
在 UniApp 开发中,如果你想自定义原生 input 组件键盘上按钮的文字,你需要利用 Vue 的 `uni.form.setFieldsValue` 方法以及事件监听来动态设置输入框的 placeholder 和 button text。这是一个大致的实现步骤:
1. 首先,在你的 Vue 组件模板中,引用输入框组件并绑定到一个变量,例如 `customInput`:
```html
<view class="custom-input">
<input ref="input" type="text" placeholder="默认文字" @focus="onFocus()" />
</view>
```
2. 然后,在 Vue 对象中定义 methods,如 `onFocus` 事件处理函数:
```javascript
export default {
data() {
return {
customInput: '请输入',
isCustomized: false, // 标记是否需要显示定制文本
};
},
methods: {
onFocus() {
this.isCustomized = true; // 当输入框聚焦时,标记为自定义模式
this.updatePlaceholder(); // 更新提示文本
},
updatePlaceholder() {
if (this.isCustomized) {
// 使用 uni.form.setFieldsValue 更新 placeholder 和 button text
this.$refs.input.formItem.placeholder = this.customInput;
} else {
this.$refs.input.formItem.placeholder = '默认文字'; // 恢复默认
}
},
},
};
```
3. 当需要改变键盘按钮文字时,比如你想将“保存”改为“确定”,可以在适当的地方调用 `updatePlaceholder` 方法,并更新 `customInput` 变量:
```javascript
// 示例:用户点击了一个切换按钮
handleSaveButtonClick() {
this.customInput = '确定';
this.updatePlaceholder();
}
```
4. 为了在失去焦点时恢复默认文字,你也可以在 `beforeDestroy` 或其他合适的生命周期钩子中添加相应操作。
注意:`uni.form.setFieldsValue` 是 UView UI 的一个特性,如果你使用的是原生的 WXML/WXSS 模式,可能需要找到对应的方法来控制输入框的提示和按钮文字。
在Vue.js的UNIApp开发环境中,如何定制原生input组件键盘上的按钮文字(例如替换为'保存'和'取消')?能详细解释并给出相关的代码示例吗?
在Vue.js的UNIApp开发环境中,如果你想要自定义原生`input`组件键盘上的按钮文字,可以利用`uni-app`的`customElement`功能以及`@focus`和`@blur`事件来动态修改键盘的行为。不过需要注意的是,这并不是直接操作输入法键盘,而是通过监听用户聚焦和失焦事件改变UI反馈。
首先,你需要创建一个自定义的`<input>`组件,并注册它作为原生组件。然后,你可以添加两个点击事件处理器,分别处理“保存”和“取消”按钮的逻辑:
```vue
<!-- custom-input.vue -->
<template>
<input ref="input" :value="currentValue" @focus="showCustomButtons" @blur="hideCustomButtons">
</template>
<script setup>
import { onBeforeUnmount } from 'vue';
// 定义当前值和按钮状态
const currentValue = ref('');
let isCustomButtonsShowing = false;
function showCustomButtons() {
if (!isCustomButtonsShowing) {
isCustomButtonsShowing = true;
// 在这里模拟添加虚拟按键,实际项目中需要根据平台API实现
document.body.appendChild(
`<div class='custom-keyboard'>
<button @click="handleSave">保存</button>
<button @click="handleCancel">取消</button>
</div>`
);
}
}
function handleSave() {
setValue('保存成功');
}
function handleCancel() {
setValue('');
}
function setValue(value) {
currentValue.value = value;
// 如果你希望清空虚拟按键,可以在这里移除它们
}
function hideCustomButtons() {
isCustomButtonsShowing = false;
// 清理虚拟按键
document.querySelector('.custom-keyboard').remove();
}
onBeforeUnmount(() => {
hideCustomButtons();
});
</script>
<style scoped>
.custom-keyboard {
position: fixed;
bottom: 0;
right: 0;
}
</style>
```
在上述代码中,我们创建了一个名为`custom-input`的组件,当`input`获得焦点时,会显示自定义的“保存”和“取消”按钮。当用户点击这些按钮时,相应的事件处理器会被触发,修改`currentValue`的值。
阅读全文
相关推荐
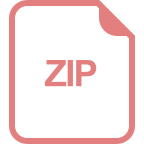
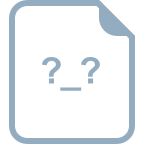

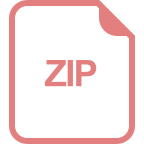
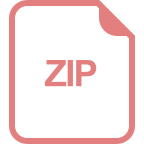
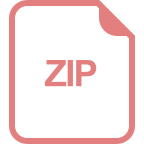
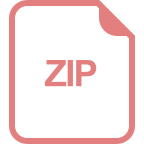
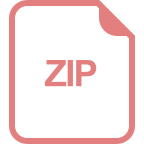
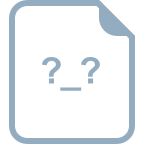
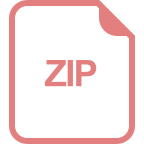
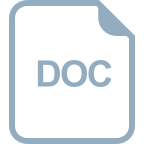
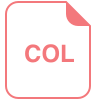


