javafx tableView一列数据中,前后相邻且数据一样的合并并显示其中一组数据怎么实现
时间: 2024-09-11 11:17:42 浏览: 104
在JavaFX中,`TableView`组件并不直接提供合并单元格的功能,因为这通常不是表格数据处理的标准操作。但是,我们可以通过一些编程技巧来模拟这种行为。实现这种效果的一种方法是在渲染表格时检测相邻单元格的数据是否相同,并且使用自定义的`TableCell`来覆盖默认的渲染行为。
以下是一个简化的示例,展示了如何为`TableView`的某一列实现相邻数据单元格合并的效果:
```java
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.stage.Stage;
public class TableViewExample extends Application {
@Override
public void start(Stage primaryStage) {
TableView<Person> table = new TableView<>();
table.setEditable(true);
TableColumn<Person, String> nameColumn = new TableColumn<>("Name");
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
// 自定义单元格工厂
nameColumn.setCellFactory(col -> new TableCell<Person, String>() {
private TextFlow textFlow = new TextFlow();
private Label label = new Label();
@Override
protected void updateItem(String item, boolean empty) {
super.updateItem(item, empty);
if (empty) {
setGraphic(null);
} else {
// 设置文本,这里可以根据需要进行格式化处理
label.setText(item);
textFlow.getChildren().clear();
textFlow.getChildren().add(label);
setGraphic(textFlow);
// 检测相邻单元格数据是否相同
int index = getIndex();
if (index > 0 && item.equals(table.getItems().get(index - 1).getName())) {
// 合并效果,这里只是简单地隐藏上一个单元格
table.getItems().get(index - 1).setName(null);
} else {
// 确保前一个单元格显示数据
table.getItems().get(index - 1).setName(item);
}
}
}
});
table.getColumns().add(nameColumn);
// 填充数据
ObservableList<Person> data = FXCollections.observableArrayList(
new Person("Alice", "UK"),
new Person("Bob", "USA"),
new Person("Alice", "USA"), // 相邻单元格内容相同
new Person("Charlie", "UK"),
new Person("Charlie", "UK") // 相邻单元格内容相同
);
table.setItems(data);
Scene scene = new Scene(table, 300, 250);
primaryStage.setTitle("合并相邻单元格示例");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
public static class Person {
private final StringProperty name;
private final StringProperty country;
public Person(String name, String country) {
this.name = new SimpleStringProperty(name);
this.country = new SimpleStringProperty(country);
}
public String getName() {
return name.get();
}
public void setName(String name) {
this.name.set(name);
}
public StringProperty nameProperty() {
return name;
}
public String getCountry() {
return country.get();
}
public void setCountry(String country) {
this.country.set(country);
}
public StringProperty countryProperty() {
return country;
}
}
}
```
在这个示例中,我们定义了一个`Person`类,并在`TableView`中创建了一个列来显示`Person`对象的`name`属性。我们通过自定义的单元格工厂来检测每一行的数据,并在相邻行数据相同的情况下隐藏前一行的数据。
请注意,这种方法只是一种视觉上的模拟,实际上并没有合并单元格。如果需要更复杂的行为,比如点击合并的单元格后只触发一次事件,可能需要进一步定制`TableCell`的行为。
阅读全文
相关推荐
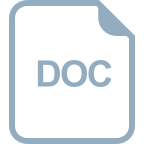
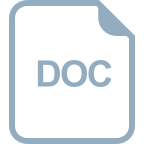
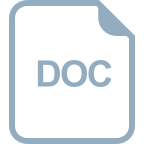
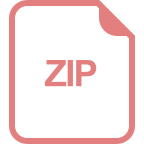
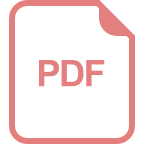
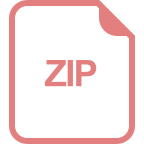
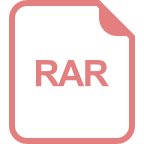
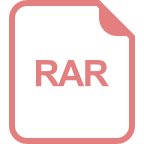
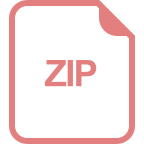
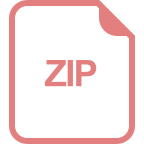
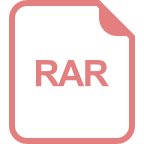
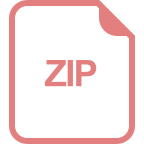
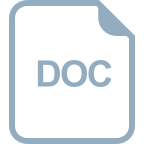
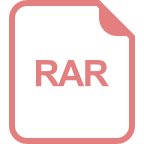
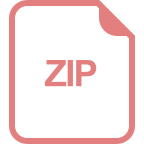
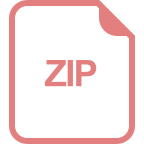