Android抽取视频所有帧,选出灰度值最大的图像,怎么操作
时间: 2024-04-14 08:30:38 浏览: 17
要抽取视频的所有帧并选择灰度值最大的图像,你可以使用 MediaMetadataRetriever 和 Bitmap 的操作来实现。
```java
MediaMetadataRetriever retriever = new MediaMetadataRetriever();
retriever.setDataSource("path_to_video_file");
String duration = retriever.extractMetadata(MediaMetadataRetriever.METADATA_KEY_DURATION);
long videoDuration = Long.parseLong(duration) * 1000; // 转换为毫秒
Bitmap maxGrayBitmap = null;
int maxGrayValue = 0;
for (long time = 0; time < videoDuration; time += 1000) {
Bitmap frame = retriever.getFrameAtTime(time, MediaMetadataRetriever.OPTION_CLOSEST);
// 计算灰度值
int grayValue = calculateGrayValue(frame);
// 更新最大灰度值和对应的图像
if (grayValue > maxGrayValue) {
maxGrayValue = grayValue;
maxGrayBitmap = frame;
}
}
// 使用 maxGrayBitmap 进行后续处理或保存
```
在上述代码中,我们使用了 `calculateGrayValue()` 方法来计算图像的灰度值。你可以根据自己的需求选择合适的算法来计算灰度值。以下是一个示例方法来计算图像的平均灰度值:
```java
private int calculateGrayValue(Bitmap bitmap) {
int width = bitmap.getWidth();
int height = bitmap.getHeight();
int totalPixels = width * height;
int sumGrayValue = 0;
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int color = bitmap.getPixel(x, y);
int red = Color.red(color);
int green = Color.green(color);
int blue = Color.blue(color);
int grayValue = (red + green + blue) / 3;
sumGrayValue += grayValue;
}
}
return sumGrayValue / totalPixels;
}
```
请注意,上述代码仅提供了一个基本的示例,并使用平均灰度值来选择最大灰度值的图像。你可以根据自己的需求选择其他方法来计算灰度值,例如最大灰度值或其他统计方法。
相关推荐
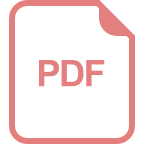
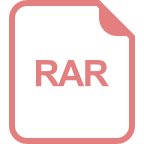














