sm2加解密c语言代码
时间: 2024-11-17 08:13:41 浏览: 3
SM2是中国的国密标准算法之一,主要用于非对称加密和数字签名。在C语言中实现SM2的加解密通常需要依赖专门的密码学库,如国家密码管理局提供的SM2开源库或者其他安全库的支持。
下面是一个简单的概述,实际操作会涉及到密钥生成、加密、解密等步骤:
```c
#include "sm2.h" // 假设这里有SM2的API头文件
// 密钥对生成
sm2_public_key_t publicKey;
sm2_private_key_t privateKey;
if (sm2_generate_keypair(&publicKey, &privateKey) != SM2_OK) {
// 错误处理...
}
// 加密(消息m通过公钥加密)
unsigned char encryptedMsg[SM2_MAX_CIPHERTEXT_LEN];
size_t len = sizeof(encryptedMsg);
if (sm2_encrypt(publicKey, plaintext, &len, encryptedMsg) != SM2_OK) {
// 错误处理...
}
// 解密(私钥解密)
unsigned char decryptedMsg[SM2_MAX_PLAINTEXT_LEN];
if (sm2_decrypt(privateKey, encryptedMsg, len, decryptedMsg) != SM2_OK) {
// 错误处理...
}
相关问题
openssl sm2加解密C语言调用
以下是 OpenSSL 库中使用 SM2 算法进行加解密的示例代码:
```c
#include <openssl/evp.h>
#include <openssl/ec.h>
#include <openssl/err.h>
#include <openssl/objects.h>
#include <openssl/sm2.h>
void sm2_encrypt_decrypt_example()
{
EVP_PKEY* pkey = NULL;
EC_KEY* ec_key = NULL;
unsigned char* plaintext = (unsigned char*)"test";
unsigned char ciphertext[1024] = {0};
int ciphertext_len = 0;
unsigned char decryptedtext[1024] = {0};
int decryptedtext_len = 0;
EVP_MD_CTX* mdctx = NULL;
// 生成 SM2 密钥对
ec_key = EC_KEY_new_by_curve_name(NID_sm2);
if (ec_key == NULL)
{
goto cleanup;
}
if (!EC_KEY_generate_key(ec_key))
{
goto cleanup;
}
pkey = EVP_PKEY_new();
if (pkey == NULL)
{
goto cleanup;
}
if (!EVP_PKEY_set1_EC_KEY(pkey, ec_key))
{
goto cleanup;
}
// 加密
mdctx = EVP_MD_CTX_new();
if (mdctx == NULL)
{
goto cleanup;
}
if (!EVP_PKEY_encrypt_init(mdctx))
{
goto cleanup;
}
if (!EVP_PKEY_CTX_set_ec_scheme(mdctx->pkey_ctx, NID_sm_scheme))
{
goto cleanup;
}
if (!EVP_PKEY_CTX_set_ec_encrypt_md(mdctx->pkey_ctx, EVP_sm3()))
{
goto cleanup;
}
if (!EVP_PKEY_CTX_set_ec_key(mdctx->pkey_ctx, ec_key))
{
goto cleanup;
}
if (!EVP_PKEY_encrypt(mdctx, ciphertext, &ciphertext_len, plaintext, strlen((char*)plaintext)))
{
goto cleanup;
}
// 解密
if (!EVP_PKEY_decrypt_init(mdctx))
{
goto cleanup;
}
if (!EVP_PKEY_CTX_set_ec_scheme(mdctx->pkey_ctx, NID_sm_scheme))
{
goto cleanup;
}
if (!EVP_PKEY_CTX_set_ec_decrypt_md(mdctx->pkey_ctx, EVP_sm3()))
{
goto cleanup;
}
if (!EVP_PKEY_CTX_set_ec_key(mdctx->pkey_ctx, ec_key))
{
goto cleanup;
}
if (!EVP_PKEY_decrypt(mdctx, decryptedtext, &decryptedtext_len, ciphertext, ciphertext_len))
{
goto cleanup;
}
printf("Decrypted text is: %s\n", decryptedtext);
cleanup:
if (mdctx != NULL)
{
EVP_MD_CTX_free(mdctx);
}
if (pkey != NULL)
{
EVP_PKEY_free(pkey);
}
if (ec_key != NULL)
{
EC_KEY_free(ec_key);
}
}
```
该示例代码中使用了 OpenSSL 库中的 `EVP_PKEY_encrypt()` 和 `EVP_PKEY_decrypt()` 函数进行加解密操作。SM2 算法的相关参数通过 `EVP_PKEY_CTX_set_ec_scheme()` 和 `EVP_PKEY_CTX_set_ec_encrypt_md()` 等函数设置。请注意,此示例代码仅作为参考,具体实现可能需要根据应用场景进行调整。
c语言 使用SM2加解密
SM2是国密算法中的一种非对称加密算法,可以用于数字证书、数字签名、密钥交换等场景。在C语言中,可以使用OpenSSL库来实现SM2加解密。
以下是一个使用OpenSSL库实现SM2加解密的示例代码:
```c
#include <openssl/ec.h>
#include <openssl/ecdsa.h>
#include <openssl/evp.h>
#include <openssl/rand.h>
void sm2_encrypt(unsigned char *in, size_t inlen, unsigned char *out, size_t *outlen, EC_KEY *ec_key) {
EVP_PKEY *pkey = EVP_PKEY_new();
EVP_PKEY_set1_EC_KEY(pkey, ec_key);
EVP_PKEY_CTX *pctx = EVP_PKEY_CTX_new(pkey, NULL);
EVP_PKEY_encrypt_init(pctx);
EVP_PKEY_CTX_set_ec_padding(pctx, EVP_ECIES_NIST);
size_t outlen_temp = *outlen;
EVP_PKEY_encrypt(pctx, out, &outlen_temp, in, inlen);
*outlen = outlen_temp;
EVP_PKEY_CTX_free(pctx);
EVP_PKEY_free(pkey);
}
void sm2_decrypt(unsigned char *in, size_t inlen, unsigned char *out, size_t *outlen, EC_KEY *ec_key) {
EVP_PKEY *pkey = EVP_PKEY_new();
EVP_PKEY_set1_EC_KEY(pkey, ec_key);
EVP_PKEY_CTX *pctx = EVP_PKEY_CTX_new(pkey, NULL);
EVP_PKEY_decrypt_init(pctx);
EVP_PKEY_CTX_set_ec_padding(pctx, EVP_ECIES_NIST);
size_t outlen_temp = *outlen;
EVP_PKEY_decrypt(pctx, out, &outlen_temp, in, inlen);
*outlen = outlen_temp;
EVP_PKEY_CTX_free(pctx);
EVP_PKEY_free(pkey);
}
int main() {
EC_KEY *ec_key = EC_KEY_new_by_curve_name(NID_sm2);
EC_KEY_generate_key(ec_key);
unsigned char plaintext[] = "hello world";
size_t plaintext_len = sizeof(plaintext);
unsigned char ciphertext[1024];
size_t ciphertext_len = sizeof(ciphertext);
sm2_encrypt(plaintext, plaintext_len, ciphertext, &ciphertext_len, ec_key);
unsigned char decrypted[1024];
size_t decrypted_len = sizeof(decrypted);
sm2_decrypt(ciphertext, ciphertext_len, decrypted, &decrypted_len, ec_key);
printf("plaintext: %s\n", plaintext);
printf("ciphertext: %s\n", ciphertext);
printf("decrypted: %s\n", decrypted);
EC_KEY_free(ec_key);
return 0;
}
```
需要注意的是,在使用OpenSSL库之前需要先安装和配置好OpenSSL库。此外,为了保证安全性,还需要对加密密钥进行严格的保护和管理。
阅读全文
相关推荐
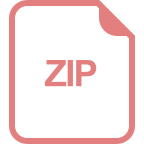
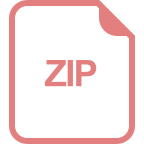
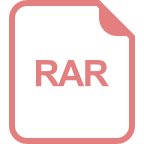
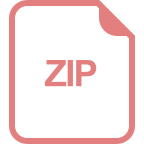
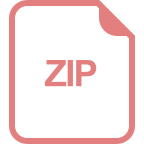






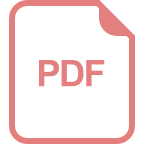
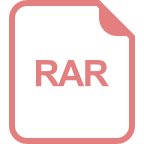
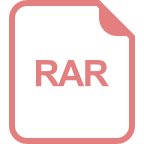