无序数组查找最优算法
时间: 2023-12-08 09:34:12 浏览: 65
无序数组的查找算法有很多种,其中一种常见的算法是线性查找。线性查找算法从数组的第一个元素开始逐个比较,直到找到目标元素或者遍历完整个数组。这种算法的时间复杂度是O(n),其中n是数组的大小。所以,线性查找算法是一种较为简单但不是最优的无序数组查找算法。
其他一些更高效的无序数组查找算法包括二分查找算法和哈希表查找算法。二分查找算法要求数组已经按照升序或降序排列,通过对比目标元素和数组中间元素的大小来确定目标元素可能出现的位置范围,然后逐步缩小范围直到找到目标元素或范围为空。二分查找算法的时间复杂度是O(log n)。而哈希表查找算法通过将数组中的元素映射到哈希表中,可以在平均情况下实现O(1)的查找时间复杂度。
综上所述,无序数组的最优查找算法取决于具体的应用场景和需求。如果数组已经有序,可以考虑使用二分查找;如果对时间复杂度有较高要求,可以考虑使用哈希表查找。但如果数组规模较小或者没有特殊要求,线性查找也是一个简单实用的选择。
相关问题
2个无序数组相同元素的数量 算法
可以使用哈希表(Hash Table)来解决这个问题。
首先,创建一个哈希表,遍历第一个数组,将数组中的每个元素作为键(key),并将其值(value)设为1。接着,遍历第二个数组,对于每个元素,查找哈希表中是否存在该元素作为键的项,如果存在,则将该项的值加1,并将该元素从第二个数组中删除。最后,哈希表中所有键的值的总和就是两个数组中相同元素的数量。
下面是该算法的Python实现代码:
```python
def count_same_elements(arr1, arr2):
hash_table = {}
count = 0
for num in arr1:
hash_table[num] = 1
for num in arr2:
if num in hash_table and hash_table[num] == 1:
count += 1
hash_table[num] = 0
return count
```
该算法的时间复杂度为O(n),其中n为两个数组中元素的总数。
数据结构创建无序数组
以下是使用Java语言创建无序数组的示例代码:
```java
public class UnorderedArray {
private float[] arr;
private int nElems;
public UnorderedArray(int max) {
arr = new float[max];
nElems = 0;
}
public void insert(float value) {
arr[nElems] = value;
nElems++;
}
public boolean delete(float value) {
int i;
for (i = 0; i < nElems; i++) {
if (arr[i] == value) {
break;
}
}
if (i == nElems) {
return false;
} else {
for (int j = i; j < nElems - 1; j++) {
arr[j] = arr[j + 1];
}
nElems--;
return true;
}
}
public boolean find(float value) {
int i;
for (i = 0; i < nElems; i++) {
if (arr[i] == value) {
break;
}
}
if (i == nElems) {
return false;
} else {
return true;
}
}
public void display() {
for (int i = 0; i < nElems; i++) {
System.out.print(arr[i] + " ");
}
System.out.println();
}
public static float[] randSort(int min, int max, int seed, float[] arr) {
Random rand = new Random(seed);
for (int i = 0; i < arr.length; i++) {
arr[i] = rand.nextInt((max - min) + 1) + min;
}
return arr;
}
}
```
以上代码中,我们定义了一个UnorderedArray类,其中包含了插入、删除、查找和展示数组等方法。我们还提供了一个randSort方法,用于生成随机的无序数组。
使用以下代码可以创建两个无序数组:
```java
float[] arr1 = UnorderedArray.randSort(1, 20, 1000, new float[20]);
float[] arr2 = UnorderedArray.randSort(1, 20, 0, new float[20]);
```
其中,arr1和arr2分别是两个无序数组,每个数组包含20个元素,元素的值在1到20之间随机生成。
相关推荐
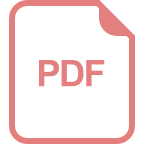
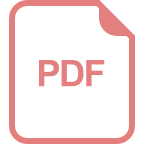
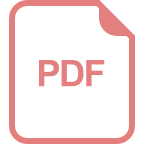












