我使用的dev c++是5.11版本,请给出贴切版本的C语言代码
时间: 2024-09-27 08:07:13 浏览: 35
对于Dev C++ 5.11版本的C语言代码编写,由于它基于GCC 4.x系列,这里依然提供一个简洁的最接近点对问题的例子,不过为了兼容,我会尽量避免使用现代特性。以下是适用于Dev C++ 5.11的C代码:
```c
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#include <time.h>
// 定义结构体存放点的坐标
typedef struct Point {
int x, y;
} Point;
// 计算两点间的距离
double distance(Point* p1, Point* p2) {
return sqrt(pow(p2->x - p1->x, 2) + pow(p2->y - p1->y, 2));
}
// 冒泡排序函数用于比较并交换点的位置
void bubble_sort(Point points[], int n) {
for (int i = 0; i < n - 1; ++i) {
for (int j = 0; j < n - i - 1; ++j) {
if (distance(&points[j], &points[j + 1]) > distance(&points[j + 1], &points[j])) {
Point temp = points[j];
points[j] = points[j + 1];
points[j + 1] = temp;
}
}
}
}
// 寻找最接近的点对
Point* find_closest_pair(Point points[], int n) {
bubble_sort(points, n);
double min_dist = INFINITY;
Point* closest_pair = NULL;
for (int i = 0; i < n - 1; ++i) {
double dist = distance(&points[i], &points[i + 1]);
if (dist < min_dist) {
min_dist = dist;
closest_pair = &points[i];
}
}
return closest_pair;
}
int main() {
srand(time(0)); // 设置随机数种子
int num_points = 5; // 临时设置点的数量,可以自定义
Point points[num_points];
// 假设这里生成一些随机点...
for (int i = 0; i < num_points; ++i) {
points[i].x = rand() % 100;
points[i].y = rand() % 100;
}
Point* closest = find_closest_pair(points, num_points);
if (closest != NULL) {
printf("The closest pair is at (%d, %d) and (%d, %d) with a distance of %.2f\n",
closest->x, closest->y,
closest->x, closest->y, distance(closest, closest + 1));
} else {
printf("No other points found. The closest pair is the same point.\n");
}
return 0;
}
```
注意,这个例子中的`INFINITY`并不是标准库里的常量,你可以用`DBL_MAX`替换。同时,对于大型数据集,上述冒泡排序效率较低,可以考虑使用其他高效算法,如分治法。
阅读全文
相关推荐
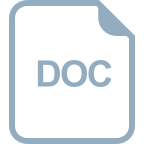
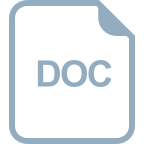
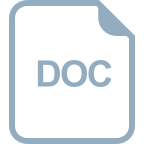
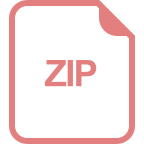
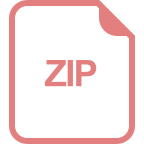
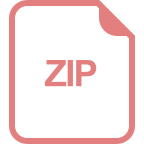
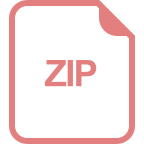
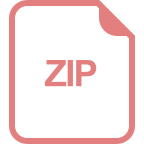
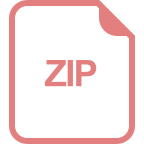
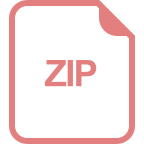
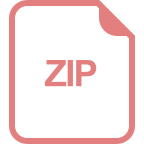
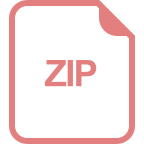
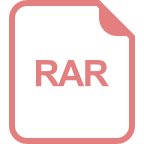
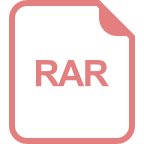


