std::map<std::string
时间: 2023-11-18 21:54:33 浏览: 100
std::map<std::string是C++ STL中的一个关联容器,它提供了一种将键映射到值的方式。其中,键和值都可以是任何类型,但键必须是唯一的。std::map<std::string>中的元素按照键的大小自动排序,并且可以通过键快速查找值。在std::map<std::string>中,每个元素都是一个键值对,其中键和值可以是任何类型,但键必须是可比较的。std::map<std::string>提供了许多方法来操作元素,例如插入、删除、查找等。可以使用迭代器来遍历std::map<std::string>中的元素。在C++11中,可以使用初始化列表来初始化std::map<std::string>,也可以使用迭代器将一个std::map<std::string>复制到另一个std::map<std::string>中。
相关问题
std::map<std::string, std::map<std::string, std::string> >::iterator it;
这是一个C++中使用`std::map`的迭代器声明示例。`std::map`是一个关联容器,用于存储键值对,并根据键进行排序。迭代器用于遍历`std::map`中的元素。
在这个示例中,`std::map`的键是`std::string`类型,值是另一个`std::map<std::string, std::string>`类型。因此,`it`被声明为一个迭代器,用于遍历这个嵌套的`std::map`结构。
具体用法如下:
```cpp
std::map<std::string, std::map<std::string, std::string>> myMap;
// 假设myMap已经包含了一些键值对
// 使用迭代器进行遍历
std::map<std::string, std::map<std::string, std::string>>::iterator it;
for (it = myMap.begin(); it != myMap.end(); ++it) {
// 访问外层map的键
std::string outerKey = it->first;
// 访问内层map的键值对
std::map<std::string, std::string> innerMap = it->second;
std::map<std::string, std::string>::iterator innerIt;
for (innerIt = innerMap.begin(); innerIt != innerMap.end(); ++innerIt) {
std::string innerKey = innerIt->first;
std::string value = innerIt->second;
// 执行相应操作
}
}
```
使用迭代器可以逐个访问和操作`std::map`中的键值对。在示例中,外层迭代器`it`用于遍历外层`std::map`的键,内层迭代器`innerIt`用于遍历内层`std::map`的键值对。
std::map<std::string, std::map<std::string, std::string> >settings_;
这是一个std::map类型的变量,它的键是std::string类型,值是另一个std::map类型的变量,该变量的键也是std::string类型,值是std::string类型。这个变量名为settings_。这个变量可以用来存储一些设置信息,例如一个程序的配置信息等。
以下是一个示例代码,演示如何使用std::map<std::string, std::map<std::string, std::string> >类型的变量:
```cpp
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, std::map<std::string, std::string> > settings_;
// 添加一些设置信息
settings_["user"]["name"] = "John";
settings_["user"]["email"] = "john@example.com";
settings_["window"]["title"] = "My App";
settings_["window"]["width"] = "800";
settings_["window"]["height"] = "600";
// 输出一些设置信息
std::cout << "User name: " << settings_["user"]["name"] << std::endl;
std::cout << "Window title: " << settings_["window"]["title"] << std::endl;
std::cout << "Window size: " << settings_["window"]["width"] << "x" << settings_["window"]["height"] << std::endl;
return 0;
}
```
阅读全文
相关推荐
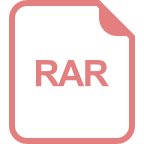
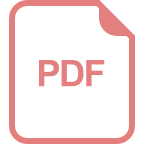
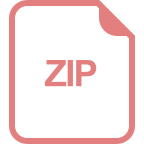













