用c++语言实现上述要求
时间: 2024-12-17 07:29:25 浏览: 1
在C++中,我们可以使用`vector`来代替Python的数组,并使用结构体或者类来表示学生信息。以下是满足要求的C++代码实现:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
// 定义学生信息结构
struct Student {
int seq; // 学生序号
float score; // 成绩
};
int main() {
std::vector<Student> students = {
{1, 90},
{2, 78},
{3, 65},
{4, 88},
{5, 55},
{6, 95}
};
// 计算平均成绩
double total_score = 0;
for (const auto& student : students) {
total_score += student.score;
}
double average_score = total_score / static_cast<double>(students.size());
std::cout << "平均成绩:" << average_score << std::endl;
// 打印60分以下的学生及其成绩
std::cout << "60分以下的学生及成绩:\n";
for (const auto& student : students) {
if (student.score < 60) {
std::cout << "序号:" << student.seq << ", 成绩:" << student.score << '\n';
}
}
// 找出85分以上的学生并按成绩排序
std::cout << "\n85分以上的学生(按成绩升序):\n";
std::vector<Student> high_scores = {student for (const auto& student : students) if student.score > 85};
std::sort(high_scores.begin(), high_scores.end(), [](const Student& a, const Student& b) {
return a.score < b.score;
});
for (const auto& student : high_scores) {
std::cout << "序号:" << student.seq << ", 成绩:" << student.score << '\n';
}
return 0;
}
```
这段代码首先定义了一个`Student`结构体,包含序号和成绩两个成员。接着,我们使用`vector`来存储学生数据,并实现了求平均分、筛选低分和高分学生等功能。
阅读全文
相关推荐
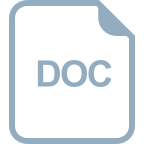
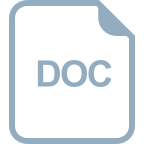
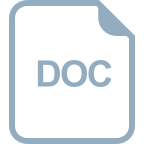
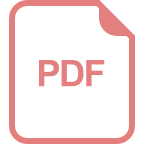
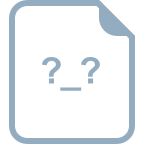
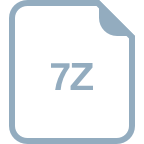
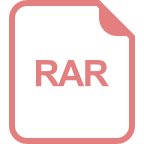
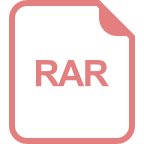
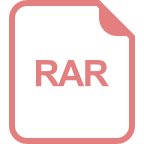
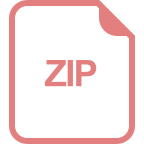
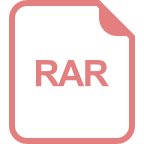
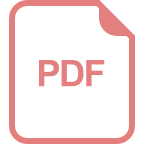
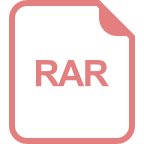
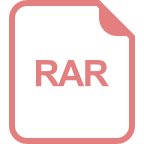
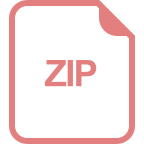
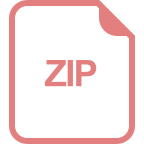
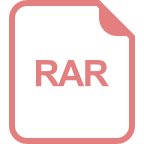
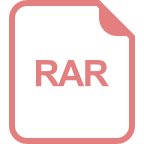
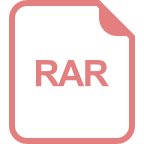