用Python绘制ROC曲线,要求可以自定义AUC值,给出详细代码
时间: 2024-04-17 15:27:34 浏览: 11
当然可以!下面是一个使用Python绘制ROC曲线的示例代码,其中可以自定义AUC值:
```python
import numpy as np
import matplotlib.pyplot as plt
def plot_roc_curve(fpr, tpr, auc):
plt.figure()
plt.plot(fpr, tpr, label='ROC curve (AUC = %0.2f)' % auc)
plt.plot([0, 1], [0, 1], 'k--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.0])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver Operating Characteristic')
plt.legend(loc="lower right")
plt.show()
def calculate_roc_curve(y_true, y_score):
sorted_indices = np.argsort(y_score)[::-1]
y_true_sorted = y_true[sorted_indices]
thresholds = np.unique(y_score)[::-1]
tpr_values = []
fpr_values = []
tp = 0
tn = 0
fp = 0
fn = 0
num_positive = np.sum(y_true)
num_negative = len(y_true) - num_positive
for threshold in thresholds:
y_pred = (y_score >= threshold).astype(int)
tp += np.sum((y_pred == 1) & (y_true_sorted == 1))
tn += np.sum((y_pred == 0) & (y_true_sorted == 0))
fp += np.sum((y_pred == 1) & (y_true_sorted == 0))
fn += np.sum((y_pred == 0) & (y_true_sorted == 1))
tpr = tp / num_positive
fpr = fp / num_negative
tpr_values.append(tpr)
fpr_values.append(fpr)
auc = np.trapz(tpr_values, fpr_values)
return fpr_values, tpr_values, auc
# 示例用法
y_true = np.array([0, 0, 1, 1, 0, 1, 0, 1])
y_score = np.array([0.2, 0.3, 0.8, 0.6, 0.4, 0.9, 0.1, 0.7])
fpr_values, tpr_values, auc = calculate_roc_curve(y_true, y_score)
plot_roc_curve(fpr_values, tpr_values, auc)
```
这段代码首先定义了一个`plot_roc_curve`函数,用于绘制ROC曲线。然后定义了一个`calculate_roc_curve`函数,用于计算ROC曲线的真阳率(True Positive Rate)和假阳率(False Positive Rate)以及AUC值。最后,示例用法给出了一个二分类问题的样例数据,并调用了上述函数进行ROC曲线的计算和绘制。
你可以根据你的需求修改`y_true`和`y_score`,以及调整其他绘图参数来绘制自定义AUC值的ROC曲线。
相关推荐










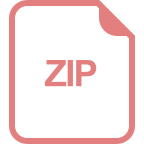
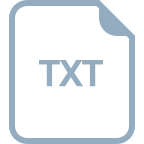
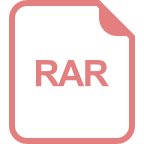