python判断字符串中是否有换行符
时间: 2023-11-02 13:05:10 浏览: 504
可以使用Python的字符串方法`find()`来判断字符串中是否包含换行符。`find()`方法返回第一个匹配的索引,如果找不到匹配项则返回-1。换行符可以用`\n`表示。
下面是一个示例代码:
```python
def has_newline(string):
if string.find('\n') != -1:
return True
else:
return False
# 测试
string1 = "This is a string without newline."
string2 = "This is a string\nwith a newline."
print(has_newline(string1)) # 输出 False
print(has_newline(string2)) # 输出 True
```
在上述代码中,`has_newline()`函数接受一个字符串作为参数,并使用`find()`方法查找换行符`\n`。如果找到换行符,则返回`True`,否则返回`False`。在测试示例中,第一个字符串没有换行符,第二个字符串包含了一个换行符。
相关问题
使用数组分别计算所输入的字符串中空格,换行符的个数
以下是Python的实现:
```python
s = input("请输入字符串:")
spaces = 0 # 空格数
newlines = 0 # 换行符数
for c in s:
if c == " ":
spaces += 1
elif c == "\n":
newlines += 1
print("空格数:", spaces)
print("换行符数:", newlines)
```
其中,`input()`函数用于从用户处获取输入的字符串;`for`循环遍历每个字符,判断是否为空格或换行符;最后输出空格数和换行符数。
python 查看字符的换行符
在 Python 中,可以使用字符串对象的 `count()` 方法来查看字符串中的某个字符出现的次数,从而判断其中换行符的数量。例如:
```python
my_string = "Hello\nworld\n!"
num_newlines = my_string.count("\n")
print(f"There are {num_newlines} newlines in the string.")
```
输出结果为:
```
There are 2 newlines in the string.
```
另外,Python 中的 `repr()` 函数可以将字符串中的特殊字符转义成可打印的形式,包括换行符。例如:
```python
my_string = "Hello\nworld\n!"
print(repr(my_string))
```
输出结果为:
```
'Hello\nworld\n!'
```
阅读全文
相关推荐
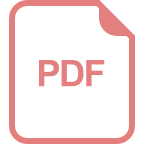
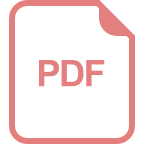








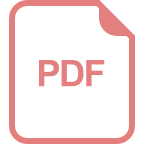
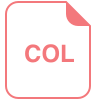
