基于遗传算法实现流水车间调度问题用python实现代码生成
时间: 2024-12-24 22:37:50 浏览: 14
基于遗传算法(Genetic Algorithm, GA)解决流水车间调度问题是优化问题的一种常见应用。这种算法模拟自然选择过程,通过迭代的方式寻找最优解。以下是用Python实现的一个简化版的遗传算法流水线调度问题的基本框架:
```python
import random
import operator
# 定义遗传算法相关的函数
def fitness_function(scheduling): # 调度方案的适应度评估函数
total_cost = calculate_total_cost(scheduling) # 计算总成本
return 1 / total_cost if total_cost else float('inf') # 较低的成本意味着更好的适应度
def crossover(parent1, parent2): # 配对交叉
start = random.randint(0, len(parent1) - 1)
end = min(start + random.randint(1, len(parent1) // 2), len(parent1))
offspring = [parent1[i] for i in range(start, end)] + parent2[end:]
offspring.extend(parent1[start:end])
return offspring
def mutation(chromosome): # 突变操作
index = random.randint(0, len(chromosome) - 1)
chromosome[index], chromosome[(index + 1) % len(chromosome)] = chromosome[(index + 1) % len(chromosome)], chromosome[index]
return chromosome
# 流水车间模型相关的类和函数
class Job:
# ... (定义任务类,包含工件ID、加工时间等)
class Factory:
def __init__(self, jobs):
self.jobs = jobs
self.schedules = []
def generate_initial_population(self, size):
# ... (生成初始种群,如随机排列的任务顺序)
def genetic_algorithm(self, population_size, generations, mutation_rate):
best_schedule = None
for _ in range(generations):
# tournament selection
parents = select_tournament(population, size=2)
child = crossover(parents[0], parents[1])
child = mutation(child) if random.random() < mutation_rate else child
# replace worst with new candidate
population = elitism(population, child)
current_best = max(population, key=fitness_function)
if not best_schedule or fitness_function(current_best) > fitness_function(best_schedule):
best_schedule = current_best
return best_schedule
# 主函数
def main():
factory = Factory(jobs_list) # 初始化工厂实例
best_schedule = factory.genetic_algorithm(
population_size, generations, mutation_rate
)
print(f"最优调度: {best_schedule}")
阅读全文
相关推荐
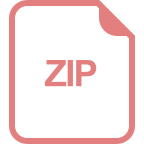
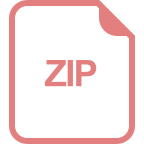
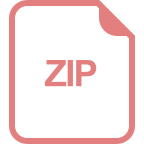
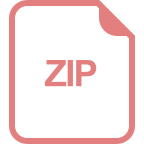
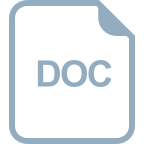
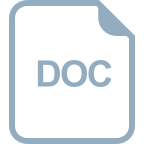
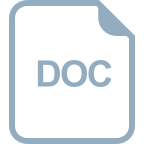
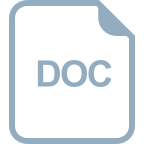


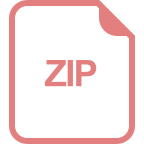
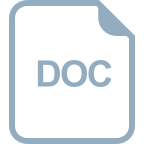
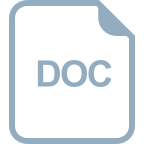
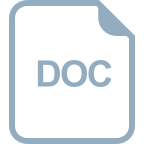


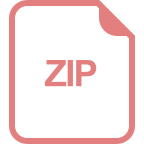

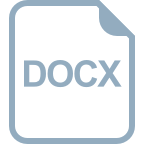