创建一个User类,属性为 id, username, age, sex。 通过Spring框架中控制反转和依赖注入,创建出 User对象,属性值为(23303150,张弘,22,男),最后通过单元测试 打印输出
时间: 2024-09-16 13:04:46 浏览: 39
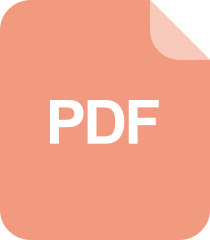
Spring Boot基础入门之基于注解的Mybatis
在Spring框架中,我们通常会创建bean来管理对象的生命周期,并通过依赖注入(Dependency Injection,DI)将bean之间的依赖关系设置好。首先,我们需要定义一个User类,然后在Spring配置文件中声明这个bean。
1. **User类定义**:
```java
import org.springframework.stereotype.Component;
@Component
public class User {
private int id;
private String username;
private int age;
private String sex;
public User(int id, String username, int age, String sex) {
this.id = id;
this.username = username;
this.age = age;
this.sex = sex;
}
// getters and setters
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public String getUsername() { return username; }
public void setUsername(String username) { this.username = username; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public String getSex() { return sex; }
public void setSex(String sex) { this.sex = sex; }
@Override
public String toString() {
return "User{" +
"id=" + id +
", username='" + username + '\'' +
", age=" + age +
", sex='" + sex + '\'' +
'}';
}
}
```
2. **Spring配置文件(applicationContext.xml 或 application.yml)**:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="user" class="com.example.User">
<constructor-arg>
<value>23303150</value>
</constructor-arg>
<constructor-arg>
<value>张弘</value>
</constructor-arg>
<constructor-arg>
<value>22</value>
</constructor-arg>
<constructor-arg>
<value>男</value>
</constructor-arg>
</bean>
</beans>
```
3. **单元测试(JUnit)**:
```java
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
@ContextConfiguration(locations = {"classpath:applicationContext.xml"})
public class UserControllerTest {
@Autowired
private User user;
@Test
public void testUser() {
System.out.println(user); // 输出User对象的toString()
assert user.getId() == 23303150;
assert user.getUsername().equals("张弘");
assert user.getAge() == 22;
assert user.getSex().equals("男");
}
}
```
运行此单元测试,你应该能看到User对象的信息被打印出来,同时也进行了属性值的断言验证。
阅读全文
相关推荐
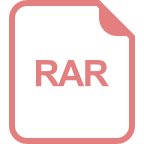
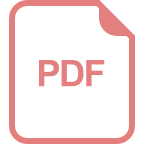
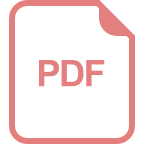
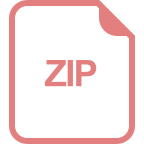
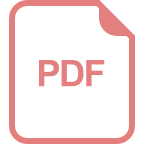
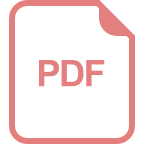
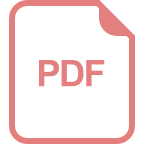
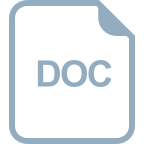
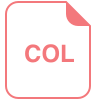


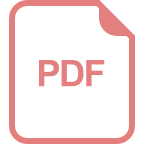
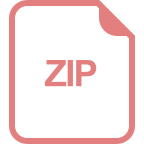
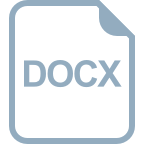