用C语言实现数据结构单链表的插入和删除
时间: 2024-09-19 16:01:58 浏览: 47
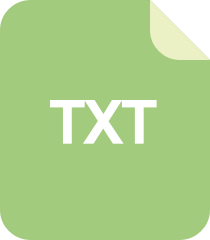
数据结构中单链表的C语言实现

在C语言中,我们可以使用结构体和指针来实现单链表的数据结构。这里简单地介绍如何实现链表的插入和删除操作:
1. 首先,定义一个链表节点的结构体,包含数据域和指向下一个节点的指针:
```c
typedef struct ListNode {
int data; // 数据域,存储整数值
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
```
2. **插入操作** (在链表末尾插入节点):
```c
void insertAtEnd(ListNode** head, int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode)); // 分配内存
if (!newNode) { // 如果分配失败,处理错误
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = NULL;
if (*head == NULL) { // 如果链表为空,直接将新节点设为首节点
*head = newNode;
} else {
ListNode* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode; // 将新节点添加到链表末尾
}
}
```
3. **删除操作** (删除给定值的第一个节点):
```c
void deleteElement(ListNode** head, int value) {
if (*head == NULL) { // 链表为空,无需删除
return;
}
if ((*head)->data == value) { // 如果头节点就是要删除的,更新头指针
ListNode* temp = *head;
*head = *head->next;
free(temp); // 释放原头节点
return;
}
ListNode* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next == NULL) { // 节点未找到,不做任何操作
return;
}
ListNode* toDelete = current->next;
current->next = current->next->next; // 删除当前节点
free(toDelete); // 释放已删除节点
}
```
阅读全文
相关推荐
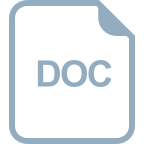
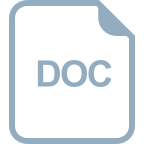

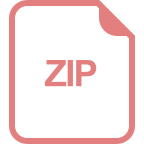
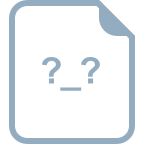
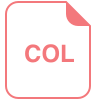
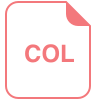



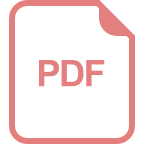
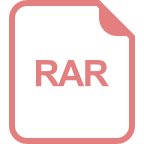
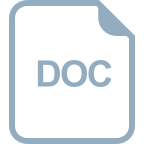
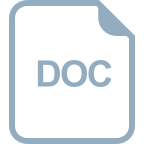



