除了 `scanf`,C++ 中有没有其他内置函数支持科学记数法的输入?
时间: 2024-10-13 12:05:09 浏览: 14
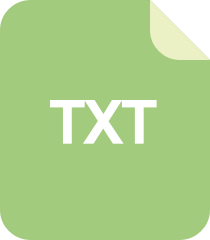
科学计数法向一般数字的转化(C语言)

在 C++ 中,虽然 `scanf` 提供了一定程度的支持,但并没有专门的内置函数直接用于科学记数法的输入。不过你可以借助 `<ios>` 和 `<sstream>` 标准库来实现自定义的输入解析。例如,你可以创建一个 `std::istringstream` 对象来逐个处理输入的字符,直到遇到错误或找到完整的科学记数法模式。下面是一个简单的示例:
```cpp
#include <iostream>
#include <sstream>
#include <string>
double scientific_notation_input(const std::string& input) {
double number = 0.0;
int exponent = 0;
std::istringstream iss(input);
std::string token;
if (!(iss >> token)) {
throw std::runtime_error("Invalid input format");
}
if (token != "e" && !iss >> number) {
throw std::runtime_error("Failed to parse base number");
}
if (!iss >> exponent) {
throw std::runtime_error("Failed to parse exponent");
}
if (iss.peek() != EOF) {
throw std::runtime_error("Extra characters after the number");
}
return number * pow(10, static_cast<double>(exponent));
}
int main() {
try {
double num = scientific_notation_input(std::cin.readline());
std::cout << "Number: " << num << " * 10^{" << num << "}\n";
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << "\n";
}
return 0;
}
```
这个函数尝试解析输入字符串,如果输入不符合预期格式,它会抛出异常。需要注意的是,这只是一个基本的例子,实际应用中可能需要额外的错误处理和验证。
阅读全文
相关推荐
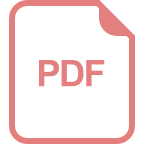
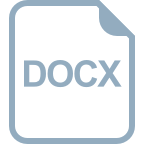















