python爬虫例子
时间: 2024-05-07 07:14:53 浏览: 29
Python 爬虫是利用 Python 编程语言编写的一种网络爬虫,可以自动化地从互联网上获取所需数据。下面是几个常见的 Python 爬虫例子:
1. 爬取豆瓣电影 Top250:使用 requests 库发起请求获取页面源码,再使用 BeautifulSoup 库进行解析,提取出电影名称、评分等信息。
2. 爬取新浪新闻:使用 requests 库获取新闻列表页的页面源码,再使用正则表达式或 BeautifulSoup 库进行解析,提取出新闻标题、时间等信息。
3. 爬取知乎热榜:使用 requests 库获取知乎热榜页面的源码,再使用 BeautifulSoup 库进行解析,提取出热门问题和对应的回答等信息。
4. 爬取淘宝商品信息:使用 Selenium 库模拟浏览器操作,获取搜索结果页面的源码,再使用正则表达式或 BeautifulSoup 库进行解析,提取出商品名称、价格、销量等信息。
5. 爬取网易云音乐歌曲:使用 requests 库获取歌曲列表页的源码,再使用 BeautifulSoup 库进行解析,提取出歌曲名称、歌手、专辑等信息。
相关问题
python 爬虫例子
以下是一些Python爬虫的例子:
1. 使用requests库进行简单的网页爬取:
```python
import requests
url = 'https://www.example.com'
response = requests.get(url)
print(response.text)
```
2. 使用BeautifulSoup库解析HTML页面:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title)
```
3. 使用Selenium库模拟浏览器行为:
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://www.example.com')
print(driver.title)
driver.quit()
```
4. 使用Scrapy框架进行高级的网页爬取:
```python
import scrapy
class MySpider(scrapy.Spider):
name = 'example'
start_urls = ['https://www.example.com']
def parse(self, response):
# 解析网页内容
title = response.css('title::text').get()
print(title)
```
5. 使用API进行数据爬取:
```python
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
data = response.json()
print(data)
```
这些例子只是爬虫的冰山一角,你可以根据自己的需求和兴趣进一步探索更多的爬虫技术和案例。
python爬虫的例子
好的,以下是一个简单的Python爬虫例子:
```
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
links = []
for link in soup.find_all('a'):
links.append(link.get('href'))
print(links)
```
这个例子使用了Python的requests和BeautifulSoup库,可以爬取一个网页中所有链接并输出。希望对你有帮助!
相关推荐
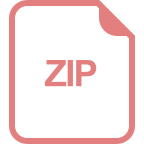
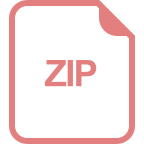
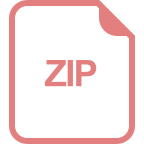












