qt中弹出文件框后点击打开如何对打开按钮进行操作
时间: 2024-09-09 19:13:33 浏览: 91
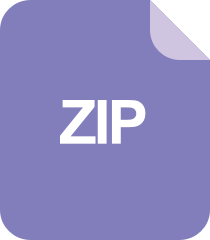
QT之打开文件对话框选择文件

在Qt中,弹出文件框通常是指使用QFileDialog类来显示一个对话框,允许用户选择文件。当你想要对点击"打开"按钮进行操作时,你通常需要使用信号和槽机制来实现。当用户点击"打开"按钮后,QFileDialog会发出一个信号,你可以连接这个信号到你自定义的槽函数,然后在槽函数中编写你需要执行的代码。
下面是一个简单的例子:
```cpp
#include <QFileDialog>
// ...
void onOpenButtonClicked()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("打开文件"), "", tr("所有文件(*.*)"));
// 在这里处理文件打开的逻辑
if (!fileName.isEmpty()) {
// 文件名不为空,表示用户已经选择了文件
// 在这里编写打开文件的代码
}
}
// ...
// 连接信号与槽
connect(openButton, &QPushButton::clicked, this, &YourClass::onOpenButtonClicked);
```
在这个例子中,`getOpenFileName`函数弹出一个文件对话框,当用户点击"打开"按钮后,会关闭对话框并返回用户选择的文件名。如果用户取消操作,返回的将是空字符串。然后你可以根据返回的文件名来执行打开文件的操作。
阅读全文
相关推荐
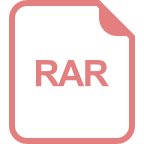
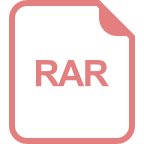

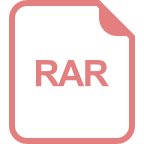
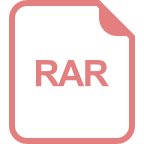
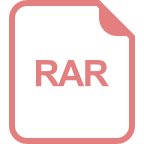
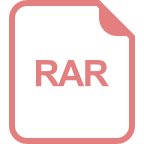
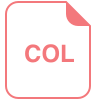









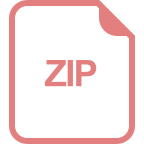