C++复制文件到系统临时文件夹temp
时间: 2024-09-06 16:06:43 浏览: 37
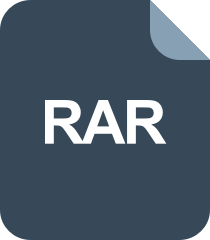
VC++ 复制文件到指定系统临时目录

在C++中,你可以使用标准库中的`std::filesystem`或者更老一些的`<fstream>`和`<cstdlib>`来进行文件操作。以下是使用`std::filesystem`复制文件到系统临时文件夹(通常路径类似`C:\Temp\`或`/tmp/`)的一个示例:
```cpp
#include <iostream>
#include <filesystem>
// 获取系统的临时目录(Windows)
std::string get_temp_directory() {
#ifdef _WIN32
char buf[MAX_PATH];
GetTempPath(MAX_PATH, buf);
return buf;
#else
char* temp_dir = std::getenv("TMPDIR");
if (temp_dir) {
return temp_dir;
} else {
return "/tmp"; // 默认Unix/Linux临时目录假设
}
#endif
}
int main() {
try {
std::string source_file = "path_to_your_file.txt";
std::string target_path = get_temp_directory() + "\\copy_of_" + std::filesystem::path(source_file).stem().string(); // 添加临时文件名前缀
// 检查目标路径是否存在,不存在则创建
if (!std::filesystem::exists(target_path)) {
std::filesystem::create_directory(std::filesystem::path(target_path).parent_path());
}
// 使用copy_file函数复制文件
std::ifstream src(source_file, std::ios::binary);
std::ofstream dst(target_path, std::ios::binary);
dst << src.rdbuf();
src.close();
dst.close();
std::cout << "File copied to temporary directory at: " << target_path << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
注意,这个示例假设了`source_file`是存在的,并且有权限读取它。如果需要处理异常情况,比如源文件不存在或权限问题,记得添加适当的错误检查。
阅读全文
相关推荐
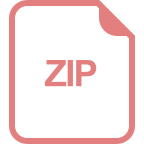
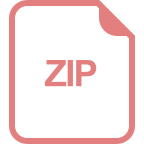


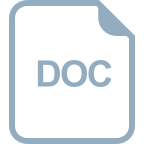
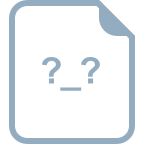
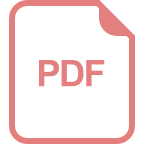
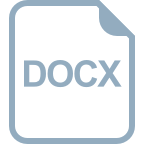
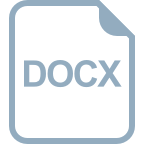
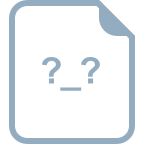
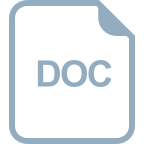
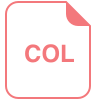
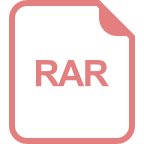
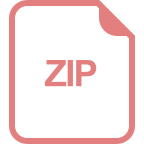
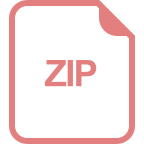
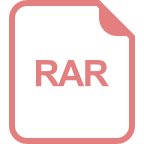
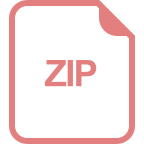