qt creator在主界面双击图片放大同时主界面消失,双击放大的图片时图片关闭返回主界面
时间: 2023-12-04 22:02:44 浏览: 143
要在Qt Creator中实现在主界面双击图片放大同时主界面消失,并在双击放大的图片时关闭图片返回主界面,您可以结合QLabel和QMainWindow来实现。下面是一个示例代码:
```cpp
#include <QApplication>
#include <QMainWindow>
#include <QLabel>
#include <QPixmap>
#include <QMouseEvent>
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr) : QMainWindow(parent) {
// 创建主界面
QLabel *imageLabel = new QLabel(this);
QPixmap pixmap("path/to/your/image.jpg"); // 替换为实际的图片路径
imageLabel->setPixmap(pixmap.scaled(400, 300, Qt::KeepAspectRatio));
imageLabel->setAlignment(Qt::AlignCenter);
setCentralWidget(imageLabel);
// 设置双击事件过滤器
imageLabel->installEventFilter(this);
}
protected:
bool eventFilter(QObject *obj, QEvent *event) override {
if (event->type() == QEvent::MouseButtonDblClick) {
QMouseEvent *mouseEvent = static_cast<QMouseEvent *>(event);
if (mouseEvent->button() == Qt::LeftButton) {
if (obj == centralWidget()) { // 主界面双击
QLabel *imageLabel = new QLabel(this);
QPixmap pixmap("path/to/your/image.jpg"); // 替换为实际的图片路径
imageLabel->setPixmap(pixmap.scaled(QApplication::desktop()->size(), Qt::KeepAspectRatio));
imageLabel->setAlignment(Qt::AlignCenter);
setCentralWidget(imageLabel);
} else { // 放大的图片双击
setCentralWidget(obj);
}
return true;
}
}
return QMainWindow::eventFilter(obj, event);
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
#include "main.moc"
```
在上述示例代码中,创建了一个MainWindow类,继承自QMainWindow。在构造函数中,创建了一个主界面,其中包含一个QLabel用于显示图片。然后,通过安装事件过滤器,在主界面和放大的图片上捕获双击事件。
在事件过滤器中,如果双击了主界面,会创建一个新的QLabel用于显示放大的图片,并将其设置为主界面的中央窗口部件。如果双击了放大的图片,会将其直接设置为主界面的中央窗口部件。
请将`path/to/your/image.jpg`替换为实际的图片路径,并根据需要调整图片的尺寸和布局。
阅读全文
相关推荐
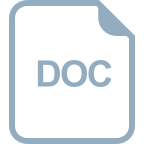
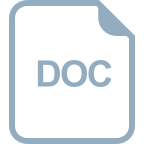
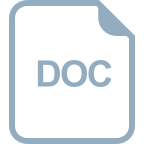

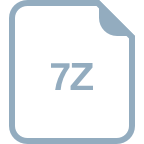
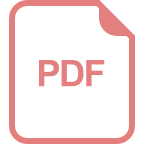
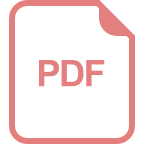
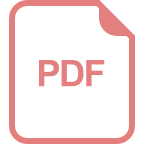
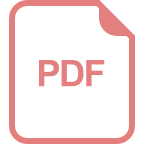
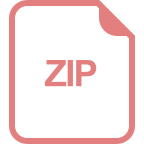
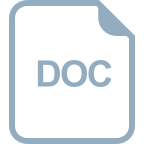
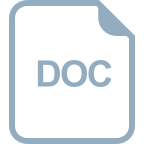
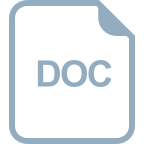
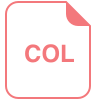

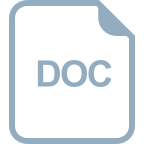
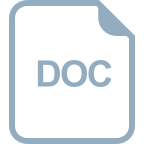
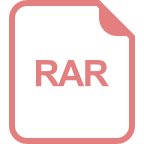
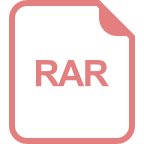