python list.find
时间: 2023-11-15 15:05:22 浏览: 147
在 Python 中,列表(list)并没有一个内置的 `.find` 方法来查找元素的索引位置。不过,你可以使用 `.index` 方法来实现类似的功能。`.index` 方法可以用来查找指定元素在列表中的第一个出现的索引位置。下面是一个示例:
```python
my_list = [1, 2, 3, 4, 5]
index = my_list.index(3)
print(index) # 输出: 2
```
在上面的例子中,`index` 变量将存储元素 `3` 在列表 `my_list` 中的索引位置,即 `2`。
需要注意的是,如果要查找的元素不在列表中,`.index` 方法将会引发一个 `ValueError` 异常。为了避免这种情况,你可以使用条件语句进行判断或者使用 `.find` 方法之前可以先判断元素是否存在于列表中:
```python
if 3 in my_list:
index = my_list.index(3)
print(index)
else:
print("元素不存在于列表中")
```
希望这可以帮助到你!如果有任何问题,请随时问我。
相关问题
Python re.findall
The `re.findall()` function is a method in Python's built-in `re` module used to search for all non-overlapping occurrences of a regular expression pattern in a given string and return a list of all matched substrings.
The syntax of `re.findall()` is as follows:
```python
re.findall(pattern, string, flags=0)
```
Here, `pattern` is the regular expression pattern to search for, `string` is the input string to search in, and `flags` (optional) is an integer value that can modify the behavior of the regular expression pattern.
The `re.findall()` function returns a list containing all the matched substrings in the order they were found in the input string. If there are no matches, an empty list is returned.
Example usage:
```python
import re
text = "The quick brown fox jumps over the lazy dog"
matches = re.findall(r"\b\w{3}\b", text)
print(matches)
# Output: ['The', 'fox', 'the', 'dog']
```
In this example, the regular expression pattern `\b\w{3}\b` searches for all three-letter words in the input string `text`. The `re.findall()` function returns a list of all matched substrings, which are the words "The", "fox", "the", and "dog".
python driver.find_element
python的driver.find_element是selenium中用于查找元素的方法之一。在使用webdriver时,可以通过不同的定位方式来查找元素,比如根据元素的id、name、xpath等等。在3.10版本下的python中,可以使用find_element_by_XX的方式来查找元素,比如find_element_by_id、find_element_by_name等等。这种方式是比较常用的,并且在百度各论坛或CSDN里也可以找到相关的资料和示例代码。
除了使用find_element_by_XX的方式,还可以使用另一种用法,即使用find_element()方法并通过参数传入定位方式和定位语句。需要导入selenium.webdriver.common.by模块中的By类。通过By类来指定定位方式,比如By.ID、By.NAME等,然后传入相应的定位语句进行查找。例如,driver.find_element(By.ID, "kw")表示通过id定位元素,定位语句为"kw"。这种方式相对更灵活一些,可以根据具体情况选择不同的定位方式。
总结起来,python的driver.find_element是selenium中用于查找元素的方法之一,在3.10版本下的python中,可以使用find_element_by_XX的方式或find_element()方法来查找元素,具体使用哪种方式可以根据实际需求来决定。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [python webdriver之find_element(python3.10版本下的变化)](https://blog.csdn.net/weixin_39407597/article/details/126394080)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [Python + Selenium 元素定位函数(find_element)](https://blog.csdn.net/m0_46243410/article/details/108504557)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
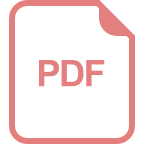



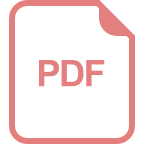
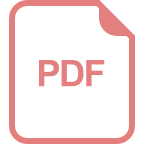
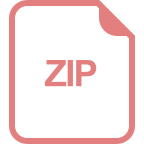
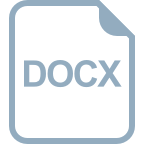
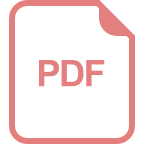
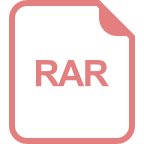
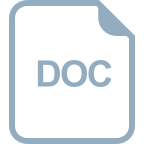




