QT地图拖拽具体实现代码
时间: 2024-09-11 14:14:13 浏览: 111
在Qt中实现地图拖拽功能,通常会使用`QGraphicsView`和`QGraphicsScene`类来处理图形视图中的拖拽交互,或者使用`QGraphicsItem`的子类来处理具体的拖拽逻辑。以下是一个简化的例子,展示如何给`QGraphicsView`添加拖拽功能:
```cpp
#include <QGraphicsView>
#include <QMouseEvent>
#include <QGraphicsScene>
class DraggableGraphicsView : public QGraphicsView {
public:
DraggableGraphicsView(QGraphicsScene* scene) : QGraphicsView(scene) {
// 设置为可以拖拽
setDragMode(QGraphicsView::ScrollHandDrag);
}
protected:
void mousePressEvent(QMouseEvent* event) override {
// 如果是左键点击,则开始拖拽
if (event->button() == Qt::LeftButton) {
dragStartPosition = event->pos();
}
QGraphicsView::mousePressEvent(event);
}
void mouseMoveEvent(QMouseEvent* event) override {
if (!(event->buttons() & Qt::LeftButton)) {
return;
}
if ((event->pos() - dragStartPosition).manhattanLength() < QApplication::startDragDistance()) {
return;
}
// 执行拖拽动作
// 这里可以获取当前鼠标下的图形项,并进行拖拽处理
QGraphicsScene* scene = this->scene();
QGraphicsItem* item = scene->itemAt(mapToScene(event->pos()), QTransform());
if (item) {
// 执行拖拽逻辑
}
QGraphicsView::mouseMoveEvent(event);
}
private:
QPoint dragStartPosition;
};
// 在主函数中使用
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QGraphicsScene scene;
DraggableGraphicsView view(&scene);
// 添加图形项到场景中
// ...
view.show();
return app.exec();
}
```
上面的代码创建了一个`DraggableGraphicsView`类,它继承自`QGraphicsView`。在这个类中重写了`mousePressEvent`和`mouseMoveEvent`方法,用来捕捉鼠标事件并执行拖拽动作。`setDragMode`方法设置视图为手形拖拽模式,允许用户通过鼠标拖动视图。
请注意,上面的代码只是一个基本的拖拽框架,具体的拖拽逻辑(如移动图形项等)需要根据实际需求来实现。如果需要拖拽的是地图组件,则可能需要更复杂的逻辑来处理地图的渲染、缩放和位置更新等。
阅读全文
相关推荐



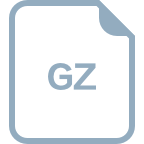


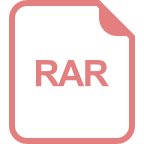












