react函数组件和类组件区别
时间: 2024-09-28 12:04:01 浏览: 45
React 的函数组件和类组件有以下几个关键区别:
1. **定义方式**[^1]:
- 函数组件是纯函数,接受props作为参数并返回JSX元素。它们没有生命周期方法,如`componentDidMount()`。只需编写一个简单的JavaScript函数即可。
```jsx
import React from 'react';
function ExampleComponent(props) {
return <h1>Hello, {props.name}</h1>;
}
```
2. **状态管理**:
- 类组件可以拥有实例状态(state),通过`this.state`来管理和更新。而函数组件不能直接修改状态,通常借助`useState` Hook来实现。
```jsx
class ExampleClass extends React.Component {
state = { count: 0 };
increment = () => this.setState({ count: this.state.count + 1 });
render() {
return <button onClick={this.increment}>Increment</button>
<p>You clicked {this.state.count} times</p>;
}
}
const FunctionalExample = (props) => {
const [count, setCount] = React.useState(0);
return (
<div>
<button onClick={() => setCount(count + 1)}>
Increment
</button>
<p>You clicked {count} times</p>
</div>
);
}
```
3. **Props和类型检查**:
- 类组件可以直接使用`propTypes`来声明prop的类型,而函数组件通常使用TypeScript进行类型检查。
```jsx
// 类组件示例
static propTypes = {
name: PropTypes.string.isRequired,
};
// 函数组件示例 (假设已安装@types/react)
type Props = {
name: string;
};
const FunctionalExample: React.FC<Props> = ({ name }) => {...};
```
4. **生命周期方法**:
- 类组件提供了一系列生命周期方法(如`componentDidMount()`),可用于初始化、更新和卸载组件。
- 函数组件不支持这些方法,但可以通过`useEffect` Hook实现相似功能。
总之,函数组件更简洁,适合小型、无状态的组件;而类组件则更适合复杂逻辑和状态管理,尽管其API更为繁琐。
阅读全文
相关推荐
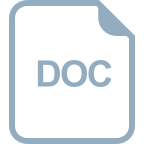
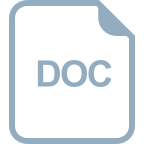
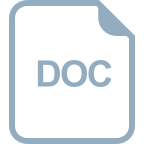








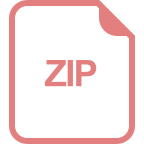
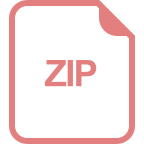
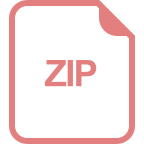


