用C语言分别实现单链表的前插法创建和后插法创建
时间: 2024-10-14 14:11:29 浏览: 51
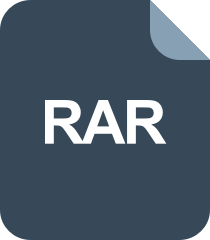
单链表 前插 后插 创建
在C语言中,我们可以使用结构体来表示链表节点,并提供函数来进行链表的前插法(在头部插入)和后插法(在尾部插入)。以下是简单的示例:
### 单链表节点定义
```c
struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
};
```
### 创建头节点(如果链表为空)
```c
struct Node* createList() {
return (struct Node*)malloc(sizeof(struct Node));
}
```
### 前插法(在链表头部插入新元素)
```c
void insertAtFront(struct Node** head_ref, int new_data) {
// 如果链表为空,直接创建一个节点
if (*head_ref == NULL) {
*head_ref = createList();
(*head_ref)->data = new_data;
(*head_ref)->next = NULL;
}
else { // 否则,在现有头部添加新的节点
struct Node* temp = *head_ref;
(*head_ref) = createList();
(*head_ref)->data = new_data;
(*head_ref)->next = temp;
}
}
```
### 后插法(在链表尾部插入新元素)
```c
void insertAtEnd(struct Node** head_ref, int new_data) {
if (*head_ref == NULL) {
*head_ref = createList();
(*head_ref)->data = new_data;
(*head_ref)->next = NULL;
}
else {
struct Node* last = *head_ref;
while (last->next != NULL)
last = last->next;
last->next = createList(); // 在最后一个节点之后插入
(*last->next)->data = new_data;
(*last->next)->next = NULL;
}
}
```
阅读全文
相关推荐
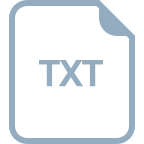
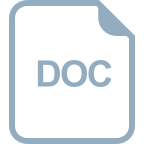
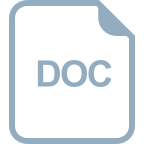
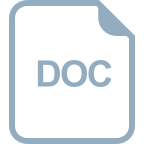
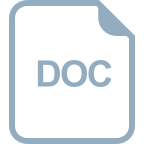
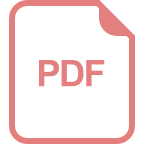
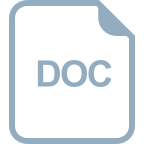
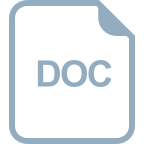
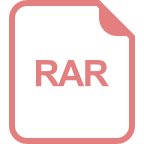
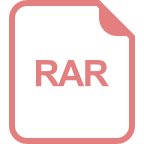