javaweb 一个jsp页面调用另一个jsp页面的double对象
时间: 2024-10-12 14:14:57 浏览: 27
在JSP (JavaServer Pages) 中,如果需要在一个JSP页面上调用并使用另一个JSP页面中的变量,通常通过模型对象(model)传递数据。首先,在包含页面(通常是父页面)中,你可以定义一个double类型的变量,并将其放入request、session或者application范围:
```jsp
<%@ page import="java.util.*" %>
<%
double value = 42.0; // 定义一个double变量值
request.setAttribute("doubleValue", value); // 将值放到request对象中
%>
```
然后,在另一个JSP页面中,你可以通过`<jsp:useBean>`标签或EL表达式从请求对象中获取这个双精度数值:
```jsp
<jsp:useBean id="dataBean" scope="request" class="java.lang.Object" />
<%= dataBean.getAttribute("doubleValue") %> // 显示double对象的值
```
这种方式可以让你在两个页面间共享数据,包括双精度对象。但是,需要注意的是,直接在JSP之间传递复杂对象可能会导致性能问题和安全风险,因此推荐在控制器层处理数据的传输。
相关问题
javaweb蛋糕商城课设 调用数据库
对于 Java Web 蛋糕商城课设,你需要使用数据库存储商品信息、订单信息和用户信息等。
1. 首先,在你的项目中添加数据库驱动包,例如 MySQL 的驱动包。
2. 在你的项目中创建一个数据库,例如命名为 cake_shop。
3. 创建数据表,例如商品表(product)、订单表(order)、用户表(user)等。
4. 在项目中编写 Java 代码,连接数据库并操作数据。
5. 例如,查询商品表中的所有商品信息,可以使用以下代码:
```
// 加载数据库驱动
Class.forName("com.mysql.jdbc.Driver");
// 获取数据库连接
String url = "jdbc:mysql://localhost:3306/cake_shop";
String username = "root";
String password = "123456";
Connection conn = DriverManager.getConnection(url, username, password);
// 执行 SQL 查询
Statement stmt = conn.createStatement();
String sql = "SELECT * FROM product";
ResultSet rs = stmt.executeQuery(sql);
// 遍历查询结果
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
double price = rs.getDouble("price");
int stock = rs.getInt("stock");
// ...
}
// 关闭连接
rs.close();
stmt.close();
conn.close();
```
6. 类似地,你可以编写 Java 代码完成添加、修改和删除商品、订单和用户等操作。
7. 最后,你可以在 JSP 页面中调用 Java 代码,将查询结果显示在页面上,以实现蛋糕商城的功能。
java web编写计算器_javaWeb 使用 jsp 和 javaBean 实现计算器功能
好的,下面是一个简单的Java Web计算器的实现过程:
1. 首先创建一个JSP页面,用于显示计算器界面。在该页面中,需要有一些按钮,如数字、运算符、清除、等于等。
2. 创建一个JavaBean类,用于处理计算器的逻辑。在该类中,需要定义一些成员变量,如操作数1、操作数2、运算符等,以及一些方法,如加、减、乘、除等。
3. 在JSP页面中,使用`<jsp:useBean>`指令引用JavaBean类,并通过`<jsp:setProperty>`指令设置JavaBean类的属性。
4. 在JSP页面中,使用`<% %>`标签引用JavaBean类中的方法,用于处理用户的操作。例如,当用户点击加号按钮时,调用JavaBean类中的加法方法。
5. 最后,使用`<%= %>`标签输出计算结果。
下面是一个简单的Java Web计算器的代码实现:
Calculator.jsp:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="com.example.bean.CalculatorBean" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Calculator</title>
</head>
<body>
<h1>Calculator</h1>
<form method="post">
<table>
<tr>
<td colspan="4"><input type="text" name="result" value="${calculatorBean.getResult()}" /></td>
</tr>
<tr>
<td><input type="button" value="7" onclick="document.forms[0].result.value += '7'" /></td>
<td><input type="button" value="8" onclick="document.forms[0].result.value += '8'" /></td>
<td><input type="button" value="9" onclick="document.forms[0].result.value += '9'" /></td>
<td><input type="button" value="+" onclick="document.forms[0].operator.value = '+'" /></td>
</tr>
<tr>
<td><input type="button" value="4" onclick="document.forms[0].result.value += '4'" /></td>
<td><input type="button" value="5" onclick="document.forms[0].result.value += '5'" /></td>
<td><input type="button" value="6" onclick="document.forms[0].result.value += '6'" /></td>
<td><input type="button" value="-" onclick="document.forms[0].operator.value = '-'" /></td>
</tr>
<tr>
<td><input type="button" value="1" onclick="document.forms[0].result.value += '1'" /></td>
<td><input type="button" value="2" onclick="document.forms[0].result.value += '2'" /></td>
<td><input type="button" value="3" onclick="document.forms[0].result.value += '3'" /></td>
<td><input type="button" value="*" onclick="document.forms[0].operator.value = '*'" /></td>
</tr>
<tr>
<td><input type="button" value="0" onclick="document.forms[0].result.value += '0'" /></td>
<td><input type="button" value="C" onclick="document.forms[0].result.value = ''" /></td>
<td><input type="submit" value="=" /></td>
<td><input type="button" value="/" onclick="document.forms[0].operator.value = '/'" /></td>
</tr>
<tr>
<td colspan="4"><input type="hidden" name="operator" /></td>
</tr>
</table>
</form>
</body>
</html>
```
CalculatorBean.java:
```java
package com.example.bean;
public class CalculatorBean {
private double operand1;
private double operand2;
private String operator;
private double result;
public CalculatorBean() {
}
public double getOperand1() {
return operand1;
}
public void setOperand1(double operand1) {
this.operand1 = operand1;
}
public double getOperand2() {
return operand2;
}
public void setOperand2(double operand2) {
this.operand2 = operand2;
}
public String getOperator() {
return operator;
}
public void setOperator(String operator) {
this.operator = operator;
}
public double getResult() {
return result;
}
public void setResult(double result) {
this.result = result;
}
public void add() {
result = operand1 + operand2;
}
public void subtract() {
result = operand1 - operand2;
}
public void multiply() {
result = operand1 * operand2;
}
public void divide() {
result = operand1 / operand2;
}
}
```
在以上代码中,我们通过`<jsp:useBean>`指令引用了CalculatorBean类,并使用`<jsp:setProperty>`指令设置了CalculatorBean类的属性。在表单提交时,我们通过JavaScript获取到用户的操作数和运算符,然后调用相应的JavaBean方法处理计算逻辑,最后通过`<%= %>`标签输出计算结果。
希望这篇文章能够帮助你实现一个简单的Java Web计算器。
阅读全文
相关推荐
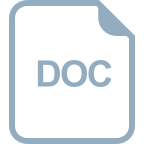
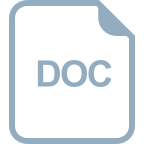
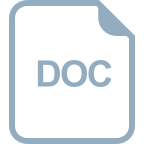
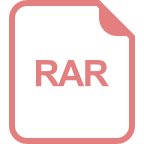
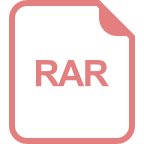
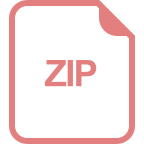
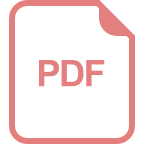
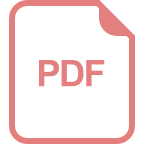
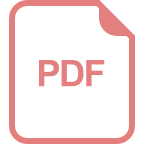
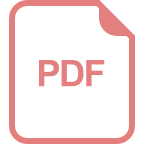
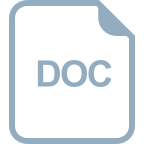





