将文件的代码改成vue3
时间: 2024-12-02 15:17:12 浏览: 4
为了将该代码迁移到 Vue 3,需要做一些必要的更改以适应新的语法和特性。以下是经过调整后的代码:
### HTML 部分(template 标签)
大部分模板部分可以直接保留,只需对绑定指令稍作调整:
```html
<template>
<div class="body">
<div class="container">
<!-- 上传区域 -->
<a-upload drag multiple :custom-request="handleUpload" :file-list="fileList" :show-upload-list="false" @change="handleChange" class="upload-area">
<div>
<a-icon type="inbox" class="upload-icon" />
<p class="upload-hint">点击或拖拽文件到这里进行上传</p>
</div>
</a-upload>
<!-- 图片列表表格 -->
<a-table :columns="columns" :data-source="imageList" row-key="id" class="image-table">
<template #customThumb="{ text, record }">
<img class="thumb-image" :src="record.thumb" @click="showImage(record.url)" />
</template>
<template #customUrl="{ text, record }">
<a :href="record.url" target="_blank" class="image-link">{{ text }}</a>
<a-button type="primary" size="small" class="download-button" @click="downloadImage(record.url)">下载</a-button>
</template>
<template #customSrcName="{ text, record }">
<div class="source-filename">{{ record.srcName }}</div>
</template>
<template #customDel="{ text, record }">
<a-popconfirm title="确定要删除吗?" ok-text="是" cancel-text="否" @confirm="deleteImage(record)">
<a-button type="danger" size="small">删除</a-button>
</a-popconfirm>
</template>
</a-table>
<!-- 按钮区域 -->
<div class="button-area">
<a-button type="primary" @click="checkTestApi" class="api-button">get测试接口</a-button>
<a-button type="primary" @click="checkTestApi2" class="api-button">post测试接口</a-button>
<a-button type="primary" @click="checkTestApi3" class="api-button">patch测试接口</a-button>
<a-button type="primary" @click="checkTestApi4" class="api-button">put测试接口</a-button>
<a-button type="primary" @click="checkTestApi5" class="api-button">delete测试接口</a-button>
</div>
<!-- 图片预览弹窗 -->
<a-modal :body-style="{ display: 'flex', justifyContent: 'center', alignItems: 'center', padding: '20px', backgroundColor: '#f9f9f9' }" v-model:visible="visible" :footer="null" :centered="true" class="image-modal">
<img alt="example" :src="selectedImageUrl" class="modal-image" />
</a-modal>
</div>
</div>
</template>
```
### JavaScript 部分(script 标签)
使用 Vue 3 的 Composition API 重构:
```javascript
<script setup>
import { ref } from 'vue';
import { message } from 'ant-design-vue';
import { testApi, uploadImage, fetchImages, testApi2, testApi3, testApi4, testApi5 } from '../utils/api';
const loading = ref(false);
const fileList = ref([]);
const imageList = ref([]);
const columns = [
{ title: '图片id', dataIndex: 'id', key: 'id' },
{ title: '图片地址', dataIndex: 'url', key: 'url', slots: { customRender: 'customUrl' } },
{ title: '缩略图', dataIndex: 'thumb', key: 'thumb', slots: { customRender: 'customThumb' } },
{ title: '源文件名', dataIndex: 'srcName', key: 'srcName', slots: { customRender: 'customSrcName' } },
{ title: '删除链接', dataIndex: 'del', key: 'del', slots: { customRender: 'customDel' } }
];
const visible = ref(false);
const selectedImageUrl = ref('');
const handleUpload = async ({ file }) => {
loading.value = true;
const formData = new FormData();
formData.append('image', file.originFileObj || file);
formData.append('token', '0ace6f265f2dd2cb1f97ac412c4ddc3e');
try {
const response = await uploadImage(formData);
if (response.code === 200) {
imageList.value.push({
id: response.id,
srcName: response.srcName,
url: response.url,
thumb: response.thumb,
del: response.del
});
} else {
console.error('上传失败', response.message);
}
} catch (error) {
console.error('请求错误', error);
} finally {
loading.value = false;
}
};
const handleChange = (info) => {
fileList.value = info.fileList;
};
const showImage = (url) => {
selectedImageUrl.value = url;
visible.value = true;
};
const downloadImage = async (url) => {
try {
const response = await fetchImages(url);
if (response && response.download_url) {
window.location.href = `http://192.168.5.125:5000${response.download_url}`;
} else {
console.error('无效的响应:', response);
}
} catch (error) {
console.error('请求错误:', error);
}
};
const deleteImage = (record) => {
imageList.value = imageList.value.filter(item => item.srcName !== record.srcName);
};
const checkApi = async (apiFunction) => {
try {
const response = await apiFunction();
if (response && response.code === 200) {
message.success(response.message + ' 接口可用', 1);
} else {
console.error('接口不可用');
}
} catch (error) {
console.error('请求错误', error);
}
};
const checkTestApi = () => checkApi(testApi);
const checkTestApi2 = () => checkApi(testApi2);
const checkTestApi3 = () => checkApi(testApi3);
const checkTestApi4 = () => checkApi(testApi4);
const checkTestApi5 = () => checkApi(testApi5);
</script>
```
### CSS 部分(style 标签)
CSS 部分保持不变:
```css
<style scoped>
.body {
width: 100%;
min-height: 740px;
background-color: #FFFFF0;
}
.ant-upload-hint {
margin-top: 8px;
}
.container {
max-width: 1400px;
margin: 0 auto;
padding: 20px;
background-color: #ffebf0;
border-radius: 15px;
box-shadow: 0 6px 12px rgba(0, 0, 0, 0.15);
font-family: 'Comic Sans MS', sans-serif;
}
.upload-area {
border: 3px dashed #ff66b2;
padding: 40px;
border-radius: 15px;
text-align: center;
background-color: #ffe6f0;
position: relative;
top: -25px;
}
.upload-icon {
font-size: 64px;
color: #ff66b2;
}
.upload-hint {
font-size: 18px;
color: #595959;
margin-top: 10px;
}
.image-table {
width: 100%;
margin-top: 20px;
background-color: #ffffff;
border-radius: 15px;
box-shadow: 0 6px 12px rgba(0, 0, 0, 0.1);
}
.thumb-image {
width: 80px;
height: 80px;
border-radius: 5px;
cursor: pointer;
}
.image-table .image-link {
width: 650px;
word-wrap: break-word;
}
.image-table .source-filename {
width: 300px;
word-wrap: break-word;
}
.image-link {
width: 376px;
color: #40a9ff;
}
.download-button {
margin-left: 10px;
}
.button-area {
display: flex;
gap: 15px;
justify-content: center;
margin-top: 20px;
}
.api-button {
width: 120px;
border-radius: 8px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.2);
}
.image-modal {
border-radius: 10px;
}
.modal-image {
max-width: 100%;
max-height: 100%;
border-radius: 8px;
object-fit: contain;
}
</style>
```
这些更改使代码兼容 Vue 3,并采用了 Composition API 来管理状态和逻辑。
阅读全文
相关推荐
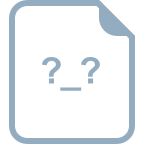
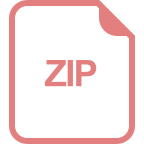
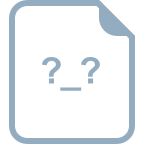















