c++ 创建文件夹桌面快捷方式
时间: 2023-08-03 12:02:18 浏览: 324
您可以使用以下代码在C++中创建文件夹和桌面快捷方式:
```cpp
#include <iostream>
#include <windows.h>
#include <shlobj.h>
int main() {
// 创建文件夹
std::string folderPath = "C:\\path\\to\\folder";
if (!CreateDirectory(folderPath.c_str(), NULL)) {
std::cout << "无法创建文件夹!" << std::endl;
return 1;
}
// 创建桌面快捷方式
std::string desktopPath;
HRESULT result = SHGetFolderPathA(NULL, CSIDL_DESKTOPDIRECTORY, NULL, 0, desktopPath);
if (result != S_OK) {
std::cout << "无法获取桌面路径!" << std::endl;
return 1;
}
std::string shortcutPath = desktopPath + "\\MyFolderShortcut.lnk";
result = CoInitialize(NULL);
if (result != S_OK) {
std::cout << "无法初始化COM库!" << std::endl;
return 1;
}
IShellLink* pShellLink;
result = CoCreateInstance(CLSID_ShellLink, NULL, CLSCTX_INPROC_SERVER, IID_IShellLink, (LPVOID*)&pShellLink);
if (result != S_OK) {
std::cout << "无法创建Shell链接实例!" << std::endl;
CoUninitialize();
return 1;
}
pShellLink->SetPath(folderPath.c_str());
pShellLink->SetDescription("My Folder Shortcut");
IPersistFile* pPersistFile;
result = pShellLink->QueryInterface(IID_IPersistFile, (LPVOID*)&pPersistFile);
if (result != S_OK) {
std::cout << "无法获取IPersistFile接口!" << std::endl;
pShellLink->Release();
CoUninitialize();
return 1;
}
result = pPersistFile->Save(shortcutPath.c_str(), TRUE);
if (result != S_OK) {
std::cout << "无法保存快捷方式!" << std::endl;
pPersistFile->Release();
pShellLink->Release();
CoUninitialize();
return 1;
}
pPersistFile->Release();
pShellLink->Release();
CoUninitialize();
std::cout << "文件夹和桌面快捷方式创建成功!" << std::endl;
return 0;
}
```
请确保将 `C:\path\to\folder` 替换为您要创建的文件夹的实际路径。这段代码使用了Windows API来创建文件夹和桌面快捷方式。
阅读全文
相关推荐
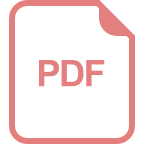
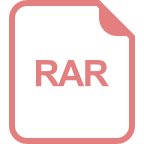

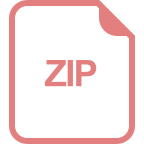
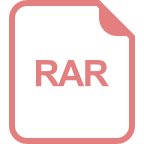
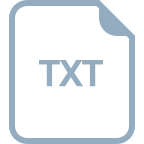
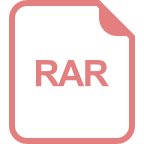
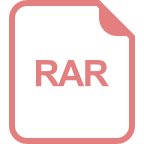
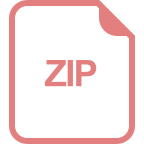
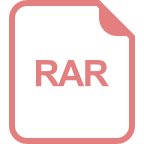
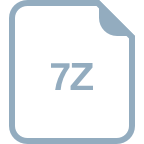
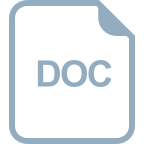
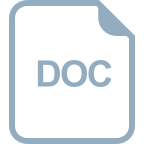
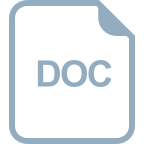
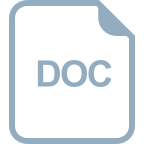
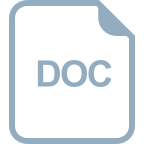