vue3子组件给父传参,父组件是setup,子组件不是steup
时间: 2024-12-15 17:12:35 浏览: 6
在 Vue 3 中,如果你的父组件使用了 `setup` 函数而不是传统的选项式编写,而子组件没有采用 `setup`,那么传递数据通常会通过 `props` 和 `ref` 来实现。在这种父子组件交互的场景下,你可以这样做:
1. **在父组件中**(setup函数内)创建一个响应式的变量,并通过 `props` 传递给子组件:
```javascript
import { ref } from 'vue'
export default {
props: {
myData: {
type: Object,
// 如果需要默认值,可以添加 default 或者 required
},
},
setup(props) {
const parentValue = ref(props.myData);
return {
parentValue,
};
},
};
```
2. **在子组件中**接受并使用这个 prop:
```html
<template>
<div>
子组件的数据:{{ $parent.parentValue }}
</div>
</template>
<script>
export default {
// 不需要 setup 函数
props: ['myData'],
};
</script>
```
相关问题
vue3子组件给父传参,父组件是setup语法糖,子组件不是steup语法糖
在Vue 3中,如果父组件使用了`setup()`函数,而子组件未使用这个新特性,你需要通过传统的方式进行通信。在这种情况下,你可以使用`props`属性从父组件向子组件传递数据。
在父组件中,创建一个`data`对象,并将需要传递的数据赋值给它,然后将这个数据作为`props`定义并传给子组件:
```javascript
// 父组件 template 和 setup 部分
<template>
<ChildComponent :myProp="parentData" />
</template>
<script setup>
import ChildComponent from './ChildComponent.vue'
const parentData = '这是父组件的数据';
</script>
```
在子组件中,接收这个prop并处理:
```vue
// 子组件 template 和 script 部分
<template>
<div>{{ myProp }}</div>
</template>
<script>
export default {
props: ['myProp'],
// 如果子组件也需要响应式数据,可以使用ref 或者 computed
};
</script>
```
vue3子组件给父传参
在 Vue3 中,子组件向父组件传递参数可以通过 emit 方法实现。具体步骤如下:
1. 在子组件中定义一个事件,并使用 emit 方法触发该事件,同时将需要传递的参数作为 emit 方法的参数传入。
```
// 子组件中
<template>
<button @click="handleClick">点击</button>
</template>
<script>
export default {
methods: {
handleClick() {
this.$emit('my-event', 'hello world')
}
}
}
</script>
```
2. 在父组件中监听该事件,并在事件处理函数中获取子组件传递的参数。
```
// 父组件中
<template>
<child-component @my-event="handleEvent"></child-component>
</template>
<script>
import ChildComponent from './ChildComponent.vue'
export default {
components: {
ChildComponent
},
methods: {
handleEvent(msg) {
console.log(msg) // 输出 'hello world'
}
}
}
</script>
```
阅读全文
相关推荐
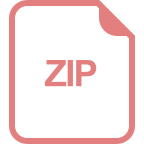
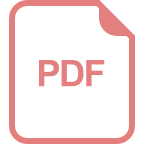
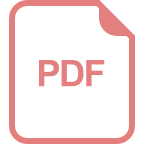













