传智会员餐厅不仅可以兑换礼品还可以获取兑奖券。顾客在支付订单时可以使用100积分抽取一次代金券,从而抵扣部分销售金额,已知顾客每次可抽取的代金券金额是0~10元随机金额,本任务要求编写一个程序实现代金券支付抵扣。java实现
时间: 2024-09-28 07:16:20 浏览: 29
在Java中实现这个功能,你可以创建一个简单的类来模拟抽签过程,并在支付方法中应用它。首先,我们可以创建一个`Coupon`类表示代金券,然后在`Customer`类里实现积分兑换和抽签的功能。以下是一个简化的示例:
```java
import java.util.Random;
// 代金券类
class Coupon {
private int value; // 代金券面额
public Coupon(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
// 客户类
public class Customer {
private int points; // 积分余额
private List<Coupon> coupons; // 存放抽到的代金券列表
public Customer(int initialPoints) {
this.points = initialPoints;
this.coupons = new ArrayList<>();
}
// 抽取代金券方法
public void drawCoupon(int maxValue) {
Random random = new Random();
int drawnValue = random.nextInt(maxValue + 1); // 生成0到10的随机数
if (drawnValue > 0) { // 如果抽到非零金额
coupons.add(new Coupon(drawnValue)); // 添加代金券
points -= drawnValue; // 减少积分
}
}
// 模拟支付并使用代金券抵扣
public double payWithCoupons(double totalAmount) {
if (coupons.isEmpty()) {
System.out.println("您还没有代金券");
return totalAmount;
} else {
double discountedAmount = totalAmount;
for (Coupon coupon : coupons) {
discountedAmount -= coupon.getValue(); // 使用每个代金券抵扣
if (discountedAmount <= 0) {
break; // 如果不足以抵扣剩余金额,则停止
}
}
return discountedAmount; // 返回实际需要支付的金额
}
}
}
// 示例用法
public static void main(String[] args) {
Customer customer = new Customer(100);
customer.drawCoupon(10); // 假设初始有100积分,抽取一次
double totalCost = 50.0;
double paid = customer.payWithCoupons(totalCost);
System.out.printf("顾客支付了%.2f元, 使用了代金券%.2f元\n", paid, customer.getCoupons().stream().mapToInt(Coupon::getValue).sum());
}
```
阅读全文
相关推荐
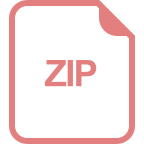
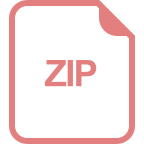
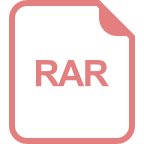

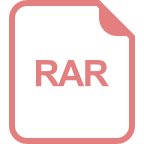
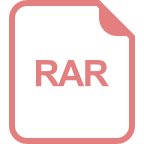
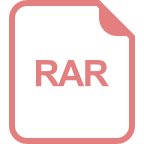
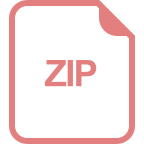
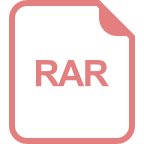
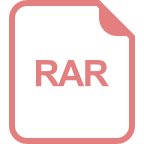
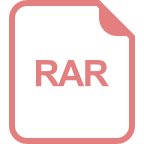
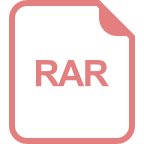
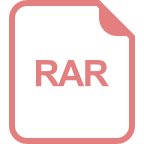
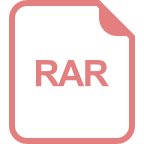
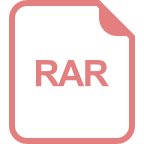
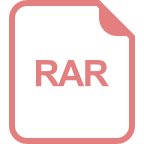
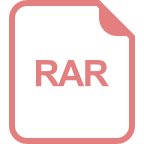