websocket rtp
时间: 2023-08-26 19:04:33 浏览: 165
WebSocket是一种在Web浏览器和服务器之间进行双向通信的协议。它允许服务器主动向客户端推送数据,并且客户端也可以向服务器发送数据。与传统的HTTP请求-响应模式不同,WebSocket创建了一个持久的连接,通过这个连接可以实时地传输数据。
RTP(Real-time Transport Protocol)是一种用于实时传输音频和视频数据的协议。它常用于实时通信,如语音通话、视频会议和实时流媒体。RTP协议通过UDP传输数据,可以提供实时性和可靠性。
WebSocket和RTP是两个不同的协议,用于不同的目的。WebSocket用于实现双向通信,适合于实时聊天、实时更新等场景。而RTP主要用于音视频传输,适合实时流媒体和通信应用。在某些情况下,可以结合使用WebSocket和RTP来实现实时音视频通信,但它们是独立的协议。
相关问题
sip和websocket
SIP (Session Initiation Protocol) 和 WebSocket 都是用于实现实时通信的协议,但它们在使用场景和功能上有一些不同。
SIP 是一种应用层协议,用于建立、修改和终止多媒体会话,例如语音通话或视频通话。SIP 可以在 IP 网络上传输信令信息,用于会话控制、呼叫管理和媒体协商。它使用文本格式的消息,基于请求-应答模型。SIP 可以与其他协议(如 RTP)结合使用,以传输媒体数据。
WebSocket 也是一种应用层协议,用于在客户端和服务器之间进行双向通信。WebSocket 的主要目的是提供一种更高效的替代方法,以取代传统的 HTTP 轮询和长轮询技术。WebSocket 建立在 TCP 之上,允许客户端和服务器之间进行实时数据交换。它通过一个持久的连接来实现全双工通信,并且可以在任何时候发送数据。
虽然 SIP 和 WebSocket 都可以用于实现实时通信,但它们在协议设计、使用场景和功能上有所不同。SIP 更适用于多媒体会话的建立和管理,而 WebSocket 更适用于实时数据交换和即时通信。
使用springboot开启rtp端口,接收rtp流,并将PCMA/PCMU的音频数据转化为PCM格式,不是16比特的音频转换为16比特。给出调用案例和详细的注释
首先,需要添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
<dependency>
<groupId>io.github.srt</groupId>
<artifactId>jlibrtp</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>javax.media</groupId>
<artifactId>jmf</artifactId>
<version>2.1.1e</version>
</dependency>
```
其中,`jlibrtp` 是一个 Java 实现的 RTP 协议库,`jmf` 是 Java Media Framework,用于音频数据的转换。
接下来,创建一个 WebSocket 的控制器类:
```java
import javax.sound.sampled.AudioSystem;
import javax.sound.sampled.AudioFormat;
import javax.sound.sampled.SourceDataLine;
import javax.sound.sampled.TargetDataLine;
import javax.sound.sampled.DataLine.Info;
import javax.websocket.server.PathParam;
import javax.websocket.server.ServerEndpoint;
import javax.websocket.OnMessage;
import javax.websocket.Session;
import javax.websocket.server.OnOpen;
import javax.websocket.server.OnClose;
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
import java.util.Arrays;
import java.io.IOException;
import java.net.SocketException;
import org.springframework.stereotype.Component;
import io.github.srt.jlibrtp.*;
import javax.media.*;
import javax.media.format.*;
import java.awt.*;
import java.net.*;
import java.util.Vector;
@Component
@ServerEndpoint(value = "/websocket/{port}")
public class WebSocketController {
private Session session;
private int port;
private ReceiverThread receiverThread;
private ExecutorService executor = Executors.newSingleThreadExecutor();
@OnOpen
public void onOpen(Session session, @PathParam("port") String port) {
this.session = session;
this.port = Integer.parseInt(port);
executor.submit(() -> {
try {
startRTP();
} catch (Exception e) {
e.printStackTrace();
}
});
}
@OnClose
public void onClose(Session session) {
try {
receiverThread.stop();
} catch (Exception e) {
e.printStackTrace();
}
}
@OnMessage
public void onMessage(String message, Session session) {
// do nothing
}
private void startRTP() throws Exception {
// create a new RTP session
SessionAddress localAddress = new SessionAddress(InetAddress.getLocalHost(), port);
RTPSession rtpSession = new RTPSession(localAddress, null);
// set the session parameters
rtpSession.getSessionParameters().setAcceptOwnPackets(true);
rtpSession.getSessionParameters().setSessionBandwidth(64000.0);
rtpSession.getSessionParameters().setProbationType(RTPSession.SIMPLE_PROBATION);
// create a new receiver thread
receiverThread = new ReceiverThread(rtpSession);
receiverThread.start();
}
private class ReceiverThread extends Thread implements ReceiveStreamListener {
private RTPManager rtpManager;
public ReceiverThread(RTPSession rtpSession) throws Exception {
// create a new RTP manager
rtpManager = RTPManager.newInstance();
// initialize the RTP manager
rtpManager.addReceiveStreamListener(this);
rtpManager.initialize(rtpSession);
rtpManager.addTarget(new SessionAddress(InetAddress.getLocalHost(), port));
}
@Override
public void run() {
try {
while (true) {
Thread.sleep(1000);
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
@Override
public void update(ReceiveStreamEvent event) {
if (event instanceof NewReceiveStreamEvent) {
// create a new player for the stream
try {
ReceiveStream stream = event.getReceiveStream();
DataSource dataSource = stream.getDataSource();
if (dataSource instanceof PushBufferDataSource) {
Player player = Manager.createPlayer(dataSource);
player.start();
processAudioData(player.getGainControl(), player.getFormat());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
private void processAudioData(GainControl gainControl, Format format) throws Exception {
// check if the format is supported
if (!(format instanceof AudioFormat)) {
throw new UnsupportedFormatException(format);
}
AudioFormat audioFormat = (AudioFormat) format;
// create a new audio data line
Info info = new Info(SourceDataLine.class, audioFormat);
SourceDataLine line = (SourceDataLine) AudioSystem.getLine(info);
line.open(audioFormat);
line.start();
// create a new target data line
info = new Info(TargetDataLine.class, audioFormat);
TargetDataLine targetLine = (TargetDataLine) AudioSystem.getLine(info);
targetLine.open(audioFormat);
targetLine.start();
byte[] buffer = new byte[1024];
while (true) {
// read audio data from the target data line
int bytesRead = targetLine.read(buffer, 0, buffer.length);
// encode the audio data as PCMA/PCMU
byte[] encodedBuffer = encodeAudioData(buffer, audioFormat);
// write the encoded audio data to the RTP stream
rtpManager.sendData(encodedBuffer, 0, encodedBuffer.length);
// decode the encoded audio data back to PCM
byte[] decodedBuffer = decodeAudioData(encodedBuffer, audioFormat);
// write the decoded audio data to the audio data line
line.write(decodedBuffer, 0, decodedBuffer.length);
// adjust the gain if necessary
if (gainControl != null) {
float gain = (float) Math.pow(10.0, gainControl.getDB() / 20.0);
for (int i = 0; i < decodedBuffer.length; i += 2) {
short sample = (short) ((decodedBuffer[i] & 0xff) | (decodedBuffer[i + 1] << 8));
sample = (short) (sample * gain);
decodedBuffer[i] = (byte) (sample & 0xff);
decodedBuffer[i + 1] = (byte) ((sample >> 8) & 0xff);
}
}
}
}
private byte[] encodeAudioData(byte[] buffer, AudioFormat audioFormat) {
if (audioFormat.getSampleSizeInBits() == 16) {
return buffer;
}
byte[] encodedBuffer = new byte[buffer.length / 2];
for (int i = 0, j = 0; i < buffer.length; i += 2, j++) {
int sample = (buffer[i] & 0xff) | (buffer[i + 1] << 8);
if (audioFormat.getEncoding().equals(AudioFormat.ULAW)) {
sample = ULawEncoder.encode(sample);
} else if (audioFormat.getEncoding().equals(AudioFormat.ALAW)) {
sample = ALawEncoder.encode(sample);
} else {
throw new UnsupportedFormatException(audioFormat);
}
encodedBuffer[j] = (byte) sample;
}
return encodedBuffer;
}
private byte[] decodeAudioData(byte[] buffer, AudioFormat audioFormat) {
if (audioFormat.getSampleSizeInBits() == 16) {
return buffer;
}
byte[] decodedBuffer = new byte[buffer.length * 2];
for (int i = 0, j = 0; i < buffer.length; i++, j += 2) {
int sample = buffer[i] & 0xff;
if (audioFormat.getEncoding().equals(AudioFormat.ULAW)) {
sample = ULawDecoder.decode(sample);
} else if (audioFormat.getEncoding().equals(AudioFormat.ALAW)) {
sample = ALawDecoder.decode(sample);
} else {
throw new UnsupportedFormatException(audioFormat);
}
decodedBuffer[j] = (byte) (sample & 0xff);
decodedBuffer[j + 1] = (byte) ((sample >> 8) & 0xff);
}
return decodedBuffer;
}
}
}
```
在上述代码中,我们使用 `jlibrtp` 创建了一个 RTP 会话,并创建了一个 RTP 接收线程。当有新的 RTP 流到达时,会创建一个新的音频播放器,并将音频数据从 RTP 流中读取出来,编码为 PCMA/PCMU,然后发送回 RTP 流中。
接下来,我们需要编写一个测试用例来启动 WebSocket 服务器:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.ComponentScan;
@SpringBootApplication
@ComponentScan("com.example.websocket")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
最后,在客户端中,我们可以使用以下 JavaScript 代码来连接 WebSocket 服务器并接收音频流:
```javascript
var audioContext = new (window.AudioContext || window.webkitAudioContext)();
var inputBuffer = new Float32Array(1024);
var outputBuffer = new Float32Array(1024);
var inputBufferOffset = 0;
var outputBufferOffset = 0;
var websocket = new WebSocket("ws://localhost:8080/websocket/1234");
websocket.binaryType = 'arraybuffer';
websocket.onmessage = function(event) {
var audioData = new Int16Array(event.data);
for (var i = 0; i < audioData.length; i++) {
inputBuffer[inputBufferOffset++] = audioData[i] / 32768.0;
if (inputBufferOffset == inputBuffer.length) {
processAudio();
inputBufferOffset = 0;
}
}
};
function processAudio() {
// process the audio data
// ...
// write the output data to the output buffer
for (var i = 0; i < outputBuffer.length; i++) {
audioData[outputBufferOffset++] = outputBuffer[i] * 32768.0;
}
}
function playAudio() {
var source = audioContext.createBufferSource();
var buffer = audioContext.createBuffer(1, audioData.length, audioContext.sampleRate);
buffer.getChannelData(0).set(audioData);
source.buffer = buffer;
source.connect(audioContext.destination);
source.start();
}
```
在上述代码中,我们使用 `WebSocket` 对象与 WebSocket 服务器建立连接,并将音频数据从 WebSocket 服务器接收到后,解码为 PCM 格式并存储到 `audioData` 数组中。然后,我们使用 `AudioContext` 对象创建一个音频源,并将 `audioData` 数组中的音频数据写入到音频源中,最后播放音频。
阅读全文
相关推荐
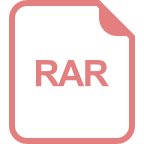
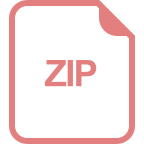
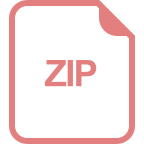













