c# 字典通过key获取value
时间: 2024-09-17 17:01:24 浏览: 61
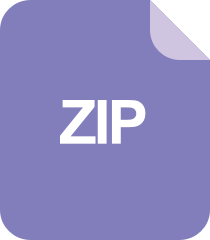
使用VC++实现的类似C#下List<Key,Value>形态的数组字典
在C#中,你可以使用Dictionary<TKey, TValue>集合结构来存储键值对。如果你想通过键(key)获取对应的值(value),可以按照以下步骤操作:
1. 首先,创建一个Dictionary实例并添加元素,例如:
```csharp
Dictionary<string, int> myDictionary = new Dictionary<string, int>();
myDictionary.Add("apple", 5);
myDictionary.Add("banana", 7);
```
2. 然后,使用`TryGetValue`方法来安全地获取值,它会返回一个包含两个元素的元组(Tuple),第一个元素是键是否存在,第二个元素是对应的值。如果键存在,`true`作为第一个元素,值作为第二个;如果键不存在则返回`false`。示例:
```csharp
int value;
if (myDictionary.TryGetValue("apple", out value))
{
Console.WriteLine($"The value of 'apple' is {value}");
}
else
{
Console.WriteLine("Key not found");
}
```
阅读全文
相关推荐
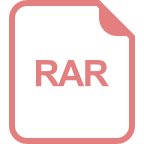
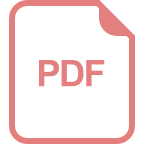
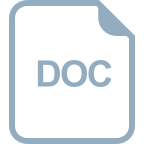













