信息增益python
时间: 2023-08-25 18:17:43 浏览: 163
在Python中计算信息增益可以使用`scikit-learn`库中的`mutual_info_classif`函数。这个函数用于计算分类问题中每个特征的信息增益。
首先,你需要导入相关的库和数据集。假设你有一个包含特征和目标变量的数据集。
```python
from sklearn.feature_selection import mutual_info_classif
import numpy as np
# 假设你的数据集是这样的
X = np.array([[1, 1, 0], [0, 0, 1], [1, 0, 1], [0, 1, 0]]) # 特征矩阵
y = np.array([1, 0, 0, 1]) # 目标变量
# 计算信息增益
info_gain = mutual_info_classif(X, y)
print(info_gain)
```
这里的`X`是特征矩阵,每行代表一个样本,每列代表一个特征。`y`是目标变量,它与特征矩阵中的样本一一对应。
`mutual_info_classif`函数将计算每个特征的信息增益,并返回一个包含信息增益值的数组。你可以根据这些信息增益值进行特征选择或其他进一步的分析。
相关问题
信息增益 python代码
好的,下面是信息增益的 Python 代码:
```python
import math
def entropy(data):
# 计算数据集的熵
num = len(data)
label_counts = {}
for feat_vec in data:
current_label = feat_vec[-1]
if current_label not in label_counts.keys():
label_counts[current_label] = 0
label_counts[current_label] += 1
entropy = 0.0
for key in label_counts:
prob = float(label_counts[key]) / num
entropy -= prob * math.log(prob, 2)
return entropy
def split_data(data, axis, value):
# 按照给定特征划分数据集
ret_data = []
for feat_vec in data:
if feat_vec[axis] == value:
reduced_feat_vec = feat_vec[:axis]
reduced_feat_vec.extend(feat_vec[axis+1:])
ret_data.append(reduced_feat_vec)
return ret_data
def choose_best_feature(data):
# 选择最好的数据集划分方式
num_features = len(data[0]) - 1
base_entropy = entropy(data)
best_info_gain = 0.0
best_feature = -1
for i in range(num_features):
feat_list = [example[i] for example in data]
unique_vals = set(feat_list)
new_entropy = 0.0
for value in unique_vals:
sub_data = split_data(data, i, value)
prob = len(sub_data) / float(len(data))
new_entropy += prob * entropy(sub_data)
info_gain = base_entropy - new_entropy
if (info_gain > best_info_gain):
best_info_gain = info_gain
best_feature = i
return best_feature
```
希望能够解答您的问题。
决策树信息增益python代码
决策树信息增益的Python代码如下所示:
```python
import math
from collections import Counter
def calc_entropy(data):
labels = [row[-1] for row in data]
label_counts = Counter(labels)
entropy = 0
for count in label_counts.values():
prob = count / len(data)
entropy -= prob * math.log2(prob)
return entropy
def calc_information_gain(data, feature_index):
feature_values = [row[feature_index] for row in data]
unique_values = set(feature_values)
entropy = calc_entropy(data)
information_gain = entropy
for value in unique_values:
sub_data = [row for row in data if row[feature_index] == value]
prob = len(sub_data) / len(data)
information_gain -= prob * calc_entropy(sub_data)
return information_gain
```
阅读全文
相关推荐
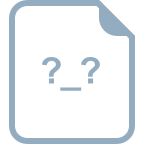
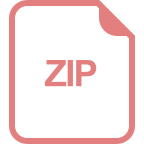
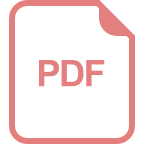
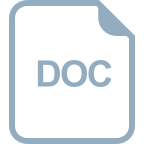
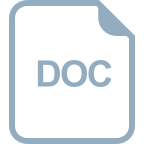
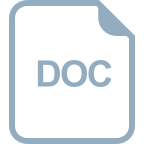
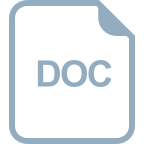
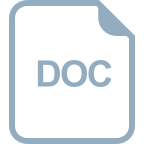






