帮我写个C#UDP广播收发
时间: 2024-12-11 11:00:36 浏览: 21
在C#中,你可以使用Socket类和UdpClient类来创建一个简单的UDP广播收发程序。以下是发送广播消息的示例:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
class UdpBroadcastSender
{
private const int PORT = 7; // UDP广播端口
private static IPEndPoint broadcastAddress = new IPEndPoint(IPAddress.Broadcast, PORT);
public static void Main()
{
try
{
using (UdpClient client = new UdpClient(PORT))
{
string message = "Hello, UDP Broadcast!";
byte[] data = Encoding.UTF8.GetBytes(message);
client.SetSocketOption(SocketOptionLevel.Socket, SocketOptionName.Broadcast, true);
client.Send(data, data.Length, broadcastAddress);
Console.WriteLine($"Sent message: {message}");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error sending broadcast: {ex.Message}");
}
}
}
```
接收广播消息的部分则需要监听来自所有网络接口的数据:
```csharp
class UdpBroadcastReceiver
{
private const int PORT = 7;
private UdpClient listener = new UdpClient(PORT);
public static void Main()
{
try
{
listener.BeginReceive(ReceiveCallback, null);
Console.WriteLine("Listening for UDP broadcasts...");
Console.ReadKey();
}
catch (Exception ex)
{
Console.WriteLine($"Error receiving: {ex.Message}");
}
finally
{
listener.Close();
}
}
private static async void ReceiveCallback(IAsyncResult result)
{
UdpReceiveResult received = listener.EndReceive(result);
Console.WriteLine($"Received: {Encoding.UTF8.GetString(received.Buffer)}");
listener.BeginReceive(ReceiveCallback, null);
}
}
```
阅读全文
相关推荐








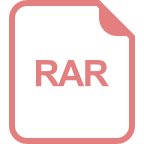






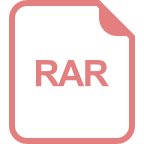


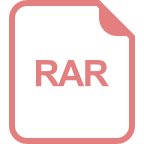